The ReactDOM Package and Its Uses
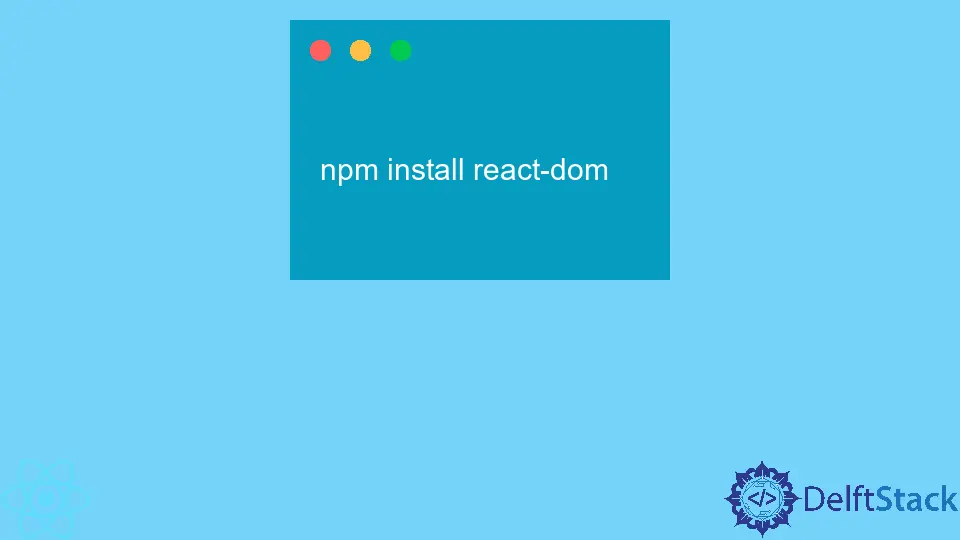
ReactDOM is one of the core libraries for building React applications. It plays a crucial role in rendering React components to the DOM, allowing developers to create dynamic and interactive user interfaces. Understanding how ReactDOM works is essential for anyone looking to harness the full potential of React.
In this article, we will explore the ReactDOM package, its primary functions, and how it integrates with React applications. Whether you’re a beginner or an experienced developer, this guide will provide insights into the practical applications of ReactDOM, making your journey through React development smoother and more efficient.
What is ReactDOM?
At its core, ReactDOM is a package that serves as the bridge between React components and the actual DOM. While React handles the component logic and state management, ReactDOM is responsible for rendering these components and updating the DOM when changes occur. This separation of concerns allows developers to focus on building reusable components without worrying about the underlying DOM manipulation.
ReactDOM provides several methods that facilitate the rendering process. The most commonly used method is ReactDOM.render()
, which takes a React element and a DOM node as arguments. This method is the starting point for rendering any React application. Additionally, ReactDOM includes methods like ReactDOM.unmountComponentAtNode()
for cleanup and ReactDOM.createPortal()
for rendering components outside the main DOM hierarchy.
Key Methods of ReactDOM
ReactDOM.render()
The ReactDOM.render()
method is the most fundamental function provided by the ReactDOM package. It allows developers to render a React component into a specific DOM node. Here’s how you can use it:
import React from 'react';
import ReactDOM from 'react-dom';
const App = () => {
return <h1>Hello, ReactDOM!</h1>;
};
ReactDOM.render(<App />, document.getElementById('root'));
Output:
Hello, ReactDOM!
In this example, we import React and ReactDOM, define a simple functional component named App
, and then render it into the DOM element with the ID of ‘root’. This method essentially takes the React component and transforms it into actual HTML that can be displayed in the browser. By using ReactDOM.render()
, you can easily mount your components to the DOM, making it the backbone of any React application.
ReactDOM.unmountComponentAtNode()
The ReactDOM.unmountComponentAtNode()
method is essential for cleaning up and removing components from the DOM. This is particularly useful when you need to unmount a component that is no longer needed, such as when navigating between views in a single-page application. Here’s how to use it:
import React from 'react';
import ReactDOM from 'react-dom';
const App = () => {
return <h1>Goodbye, ReactDOM!</h1>;
};
const rootElement = document.getElementById('root');
ReactDOM.render(<App />, rootElement);
// Later in the code, when you want to unmount:
ReactDOM.unmountComponentAtNode(rootElement);
Output:
Goodbye, ReactDOM!
In this snippet, we first render the App
component, and later, when we no longer need it, we call ReactDOM.unmountComponentAtNode(rootElement)
. This method effectively removes the component from the DOM, freeing up memory and preventing potential memory leaks. It’s a best practice to unmount components when they are no longer needed, especially in dynamic applications.
ReactDOM.createPortal()
The ReactDOM.createPortal()
method allows developers to render children into a DOM node that exists outside the hierarchy of the parent component. This is particularly useful for modals, tooltips, or any component that needs to break out of its parent’s overflow or positioning constraints. Here’s an example:
import React from 'react';
import ReactDOM from 'react-dom';
const Modal = ({ children }) => {
return ReactDOM.createPortal(
<div className="modal">
{children}
</div>,
document.getElementById('modal-root')
);
};
const App = () => {
return (
<div>
<h1>Welcome to the Portal Example</h1>
<Modal>
<h2>This is a Modal!</h2>
</Modal>
</div>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
Output:
Welcome to the Portal Example
This is a Modal!
In this example, the Modal
component uses ReactDOM.createPortal()
to render its children into a separate DOM node identified by ‘modal-root’. This allows the modal to appear above other content without being constrained by its parent component’s styles. Portals provide a powerful way to manage UI elements that require special positioning or behavior.
Conclusion
In summary, ReactDOM is an indispensable library for any React developer. Its primary methods, including ReactDOM.render()
, ReactDOM.unmountComponentAtNode()
, and ReactDOM.createPortal()
, provide essential functionality for rendering components, managing lifecycle events, and creating dynamic user interfaces. By mastering these methods, you can enhance your React applications and create engaging user experiences. As you continue to explore React, understanding how ReactDOM interacts with your components will empower you to build more efficient and effective applications.
FAQ
-
What is the purpose of ReactDOM?
ReactDOM is responsible for rendering React components to the DOM and managing updates. -
How do I unmount a component in React?
You can unmount a component using theReactDOM.unmountComponentAtNode()
method. -
What is a portal in React?
A portal is a way to render children into a DOM node that exists outside the parent component’s hierarchy. -
Can I use ReactDOM for server-side rendering?
No, ReactDOM is primarily for client-side rendering. For server-side rendering, use ReactDOMServer. -
Is ReactDOM necessary for all React applications?
Yes, ReactDOM is necessary for rendering React components to the DOM in web applications.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn