How to Use Async Syntax in the useEffect Callback
-
What Is
useEffect()
in React -
How to Use
async
Syntax WithinuseEffect
in React -
the Best Solution to Use
async
Syntax WithinuseEffect
in React
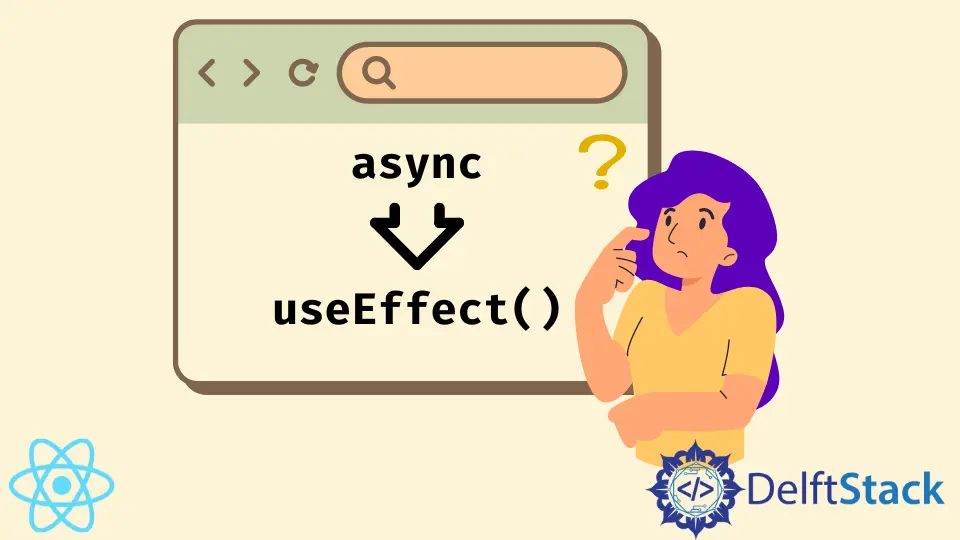
The release of React version 16.8 changed the library in many ways. With the introduction of hooks, functional components gained the ability to maintain state and handle side effects.
useEffect()
is one of the key hooks for functional components in React, and it was created specifically for handling side effects. It is fairly easy to figure out but still has some caveats that React developers should know.
This article will explore one of those caveats - how to use async
syntax within useEffect()
.
What Is useEffect()
in React
useEffect()
is a solution that allows you to handle side-effects in functional components. If you’ve previously used Class Components, you can think of it as an alternative to lifecycle methods.
The side effects in React applications should be limited to useEffect()
. Common examples include calculating time or asynchronously loading external data through requests, which brings us to our main topic.
How to Use async
Syntax Within useEffect
in React
If you try to use an async
function as a useEffect
callback, you’ll get an error saying that the hook doesn’t support it.
So what exactly can you do if you need to use this syntax for data loading or any other purpose? And why did the creators of React decide to make useEffect()
incompatible with async
syntax?
Let’s start with the second question. useEffect()
doesn’t support async
callbacks because such functions usually require cleanup.
Side-effects need to be handled delicately, and mishandling an async
function inside the hook could lead to memory leaks or other errors that could expose your application. That’s why the team behind React decided not to allow it.
As for the first question, fortunately, there’s a library that allows you to put async
functions in useEffect()
without getting an error.
the Best Solution to Use async
Syntax Within useEffect
in React
If you must use the async await
syntax, the best solution is to use a use-async-effect
package. This package provides a slightly modified useAsyncEffect()
hook, which has the same functionality as useEffect()
but allows you to use the async
syntax.
It is very easy to learn. It even accepts the same arguments as regular useEffect()
.
To learn more about installation and other details, read the official docs.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn