How to Sort Data With React-Table Library
- Understanding React-Table
- Setting Up a Basic Table
- Implementing Sorting with React-Table
- Customizing Default Sorting
- Conclusion
- FAQ
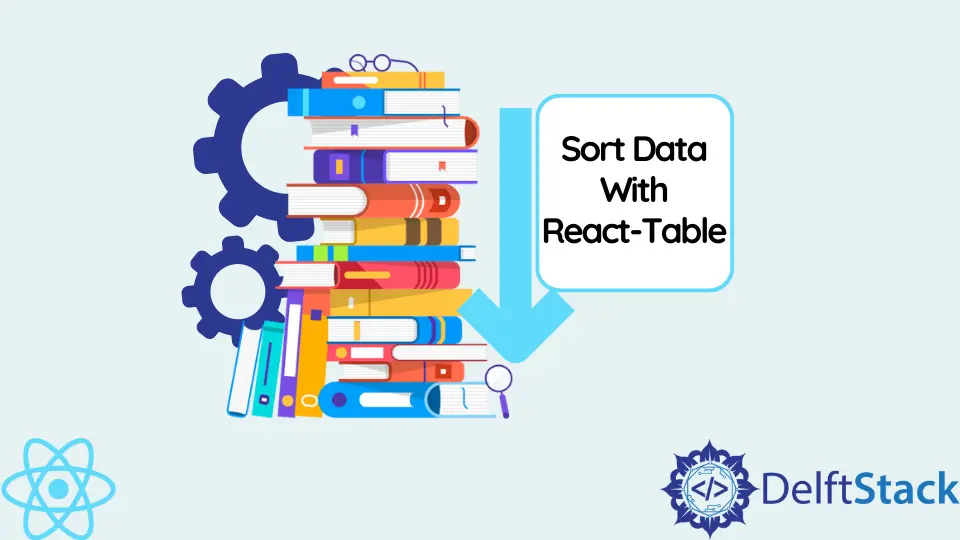
Tables are essential for presenting large volumes of data in a structured and easily digestible format. When working with data in React applications, the React-Table library stands out as a powerful tool for creating dynamic tables.
In this article, we will explore how to configure React-Table hooks to sort data by default, enhancing user experience and ensuring that your data presentation is both efficient and user-friendly. Whether you’re displaying product listings, user information, or any other type of data, mastering sorting with React-Table will elevate your application’s functionality.
Understanding React-Table
React-Table is a lightweight and flexible library that allows developers to create fully customizable tables. It provides a set of hooks that make it easy to manage table state, including sorting, filtering, and pagination. The library is designed to be easy to use while offering a high degree of customization.
To get started with React-Table, you need to install the library and set up your project. You can easily do this using npm:
npm install react-table
Once installed, you can import the necessary hooks and components from the library to create your table. The core functionality of React-Table revolves around the use of hooks like useTable
, useSortBy
, and others, which allow you to define the structure and behavior of your table.
Setting Up a Basic Table
Before diving into sorting, let’s create a basic table structure using React-Table. This will serve as the foundation for implementing sorting functionality.
import React from 'react';
import { useTable } from 'react-table';
const TableComponent = ({ columns, data }) => {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable({ columns, data });
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()}>{column.render('Header')}</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
};
export default TableComponent;
This code sets up a simple table component that accepts columns
and data
as props. The useTable
hook is used to create the table instance, which provides various properties and methods to manage the table’s behavior. The component renders the table structure, including headers and body rows.
Now that we have a basic table set up, let’s move on to implementing sorting.
Implementing Sorting with React-Table
Sorting is a crucial feature for any data table. With React-Table, you can easily add sorting capabilities using the useSortBy
hook. Below is an example of how to implement sorting in your table.
import React from 'react';
import { useTable, useSortBy } from 'react-table';
const TableComponent = ({ columns, data }) => {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable(
{ columns, data },
useSortBy
);
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps(column.getSortByToggleProps())}>
{column.render('Header')}
<span>
{column.isSorted ? (column.isSortedDesc ? ' 🔽' : ' 🔼') : ''}
</span>
</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
};
export default TableComponent;
In this code, we import the useSortBy
hook alongside useTable
. By including useSortBy
in the hook configuration, we enable sorting functionality. The getSortByToggleProps
method is used to attach sorting behavior to the header cells. Additionally, we provide visual indicators (arrows) to show the sorting direction.
When a user clicks on a column header, the table will sort the data based on that column, improving the overall data navigation experience.
Customizing Default Sorting
React-Table allows you to set default sorting when the table first loads. This can be particularly useful when you want to present data in a specific order right from the start. You can achieve this by passing a defaultSorted
property in the table configuration.
import React from 'react';
import { useTable, useSortBy } from 'react-table';
const TableComponent = ({ columns, data }) => {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable(
{ columns, data, initialState: { sortBy: [{ id: 'column_id', desc: false }] } },
useSortBy
);
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps(column.getSortByToggleProps())}>
{column.render('Header')}
<span>
{column.isSorted ? (column.isSortedDesc ? ' 🔽' : ' 🔼') : ''}
</span>
</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
};
export default TableComponent;
In this example, we set the initialState
property with a sortBy
array. The id
corresponds to the column you want to sort by default, and desc
indicates whether the sorting should be descending or ascending. This approach allows you to control how the data is displayed when the table is first rendered, providing a better user experience.
Conclusion
Sorting data in a React application can significantly enhance the user experience, especially when dealing with large datasets. The React-Table library provides a robust and flexible solution for creating sortable tables with minimal effort. By utilizing hooks like useTable
and useSortBy
, you can easily implement sorting features, customize default sorting, and create a user-friendly interface. With these tools at your disposal, you can ensure that your data is presented in an organized and efficient manner, making it easier for users to navigate and analyze the information.
FAQ
-
What is React-Table?
React-Table is a lightweight library for building dynamic tables in React applications, offering features like sorting, filtering, and pagination. -
How do I install React-Table?
You can install React-Table using npm with the commandnpm install react-table
. -
Can I customize the sorting behavior in React-Table?
Yes, React-Table allows you to customize sorting behavior, including setting default sorting when the table loads. -
Is React-Table suitable for large datasets?
Yes, React-Table is designed to handle large datasets efficiently, providing features like virtualization and lazy loading. -
How do I indicate the sorting direction in the table headers?
You can use visual indicators such as arrows or icons in the header cells to show the current sorting direction.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn