How to Custom Sort With React-Table Library
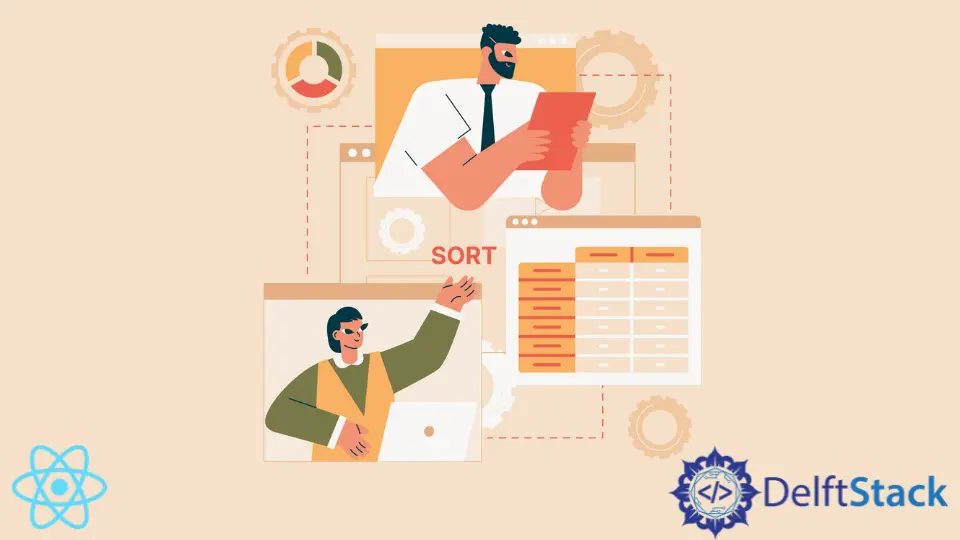
The react-table library has become a go-to tool for developers looking to create dynamic and efficient tables in React applications. With its robust features and flexibility, it allows for easy customization, including sorting.
In this article, we will dive into the intricacies of custom sorting using the react-table library. Whether you’re managing a simple dataset or a more complex table, understanding how to implement custom sorting can significantly enhance user experience and data presentation. So, let’s roll up our sleeves and explore how to make this powerful library work for you!
Understanding React-Table
Before we jump into custom sorting, let’s take a moment to understand what react-table is and why it’s widely used. React-table is a lightweight, fast, and extendable library that enables developers to create tables with sorting, filtering, and pagination capabilities. Its headless design allows for complete control over the UI and the data it displays. This flexibility is what makes it a favorite among developers.
The react-table library uses hooks, making it easy to integrate into functional components. It provides a straightforward API for adding features like sorting, which we’ll focus on in this article. By leveraging the power of hooks, you can create a table that not only displays data but also allows users to interact with it seamlessly.
Setting Up React-Table
To get started with react-table, you first need to install it in your React project. You can do this using npm or yarn. Open your terminal and run the following command:
npm install react-table
or
yarn add react-table
Once installed, you can import the necessary hooks and components from the react-table library. Here’s a basic setup to create a simple table:
import React from 'react';
import { useTable } from 'react-table';
const TableComponent = ({ columns, data }) => {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({ columns, data });
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()}>{column.render('Header')}</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
};
export default TableComponent;
Output:
A basic table structure using react-table
In this setup, we define a functional component called TableComponent
. We use the useTable
hook to create a table instance, which provides various props and methods to manage the table’s behavior. The structure includes a table header and body, where we render the data dynamically.
Implementing Custom Sorting
Now that we have a basic table, let’s implement custom sorting. React-table provides built-in sorting capabilities, but we can enhance it by creating our own sorting logic. This allows us to sort data based on custom criteria, which can be particularly useful for complex datasets.
First, let’s modify our TableComponent
to include sorting functionality. We will define a custom sorting function and apply it to our table.
const customSort = (rowA, rowB, columnId) => {
const a = rowA.values[columnId];
const b = rowB.values[columnId];
return a > b ? 1 : -1;
};
const TableComponent = ({ columns, data }) => {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({
columns,
data,
manualSortBy: true,
getSortByToggleProps: (column) => ({
onClick: () => {
const sortDirection = column.isSortedDesc ? 'asc' : 'desc';
column.toggleSortBy(sortDirection);
},
}),
sortTypes: {
custom: customSort,
},
});
return (
<table {...getTableProps()}>
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps(column.getSortByToggleProps())}>
{column.render('Header')}
{column.isSorted ? (column.isSortedDesc ? ' 🔽' : ' 🔼') : ''}
</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render('Cell')}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
};
Output:
Custom sorting functionality added to the table
In this code, we define a customSort
function that compares two rows based on a specified column. We then integrate this sorting logic into the useTable
hook by setting manualSortBy
to true and specifying our custom sort type. The getSortByToggleProps
function allows us to toggle sorting when a column header is clicked, providing visual feedback to users.
Conclusion
Custom sorting in the react-table library is a powerful feature that can significantly improve the usability of your tables. By leveraging the flexibility of this library, developers can create tables that not only display data but also allow users to interact with it in meaningful ways. Whether you’re working with simple datasets or complex information, understanding how to implement custom sorting will enhance your React applications. Now that you have the tools and knowledge, it’s time to put them into practice and create dynamic, user-friendly tables!
FAQ
-
What is react-table?
react-table is a lightweight library for creating tables in React applications, providing features like sorting, filtering, and pagination. -
How do I install react-table?
You can install react-table using npm or yarn with the commands npm install react-table or yarn add react-table. -
Can I implement custom sorting in react-table?
Yes, react-table allows for custom sorting by defining your own sorting logic and integrating it into the table configuration. -
What are the benefits of using react-table?
React-table is fast, lightweight, and offers a headless design, giving developers full control over the UI and functionality. -
How can I enhance user experience with react-table?
By implementing features like custom sorting, filtering, and pagination, you can create a more interactive and user-friendly table experience.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn