String Interpolation in React
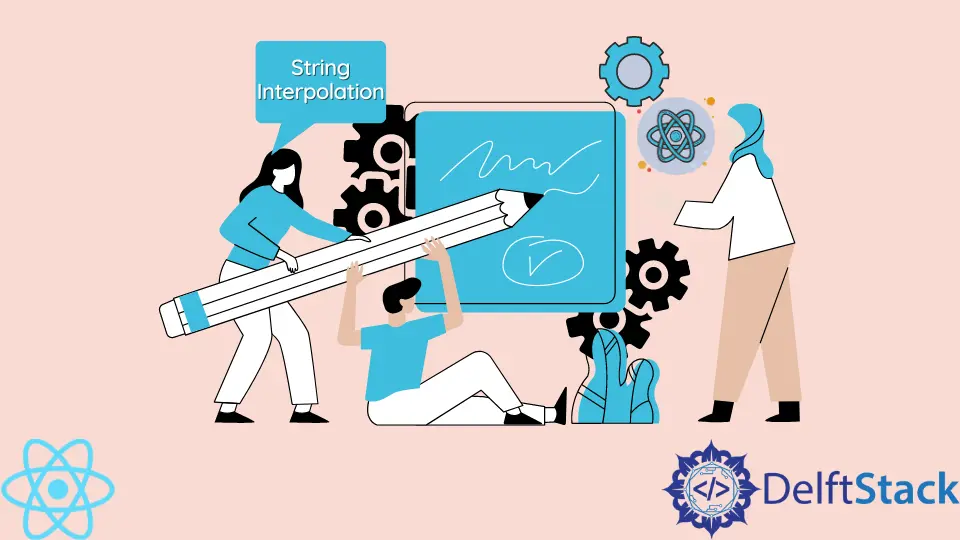
Front-end developers can use React to declaratively build visually appealing user interfaces. React code is not so simple, especially if you’re a beginner.
At some point, all React projects reach the level of complexity where they require you to combine simple values like strings and dynamic values from the state
or props
.
There are multiple ways to combine static and dynamic values in JavaScript. As luck would have it, ES6 introduced template literals, which probably provide the easiest way to combine strings and variable values.
However, it’s still possible to do string interpolation without using modern ES6 features.
React String Interpolation in ECMAScript 5 (ES5)
Let’s imagine you have a state property to store the size of the text. You might need to create a custom class by combining a regular string with the state variable.
Let’s see how to do that using traditional JavaScript.
export default function App() {
const [size, setSize] = useState("medium");
return <div className={"font-" + size}>A sample content</div>;
}
Everything goes well since we concatenate the string with a string value variable. However, even if our variable held a numeric value, JavaScript would automatically convert it to a string.
If you run this code on CodeSandbox and look into the source file, you’ll see that the class
attribute is set to the combined value.
React String Interpolation in ECMAScript 6+ (ES6+)
There’s another way to do string interpolation using template literals (also known as backticks) of modern JavaScript. This way, you can reference the entire value as one string, and you can tell JavaScript to get the variable values of specific parts.
Our example is fairly simple, so the difference might not be that impressive. But if using long strings that involve multiple variables can make a big difference.
Let’s look at an example.
export default function App() {
const [size, setSize] = useState("medium");
return <div className={`font- + ${size}`}>A sample content</div>;
}
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn