How to Sort an Array in React
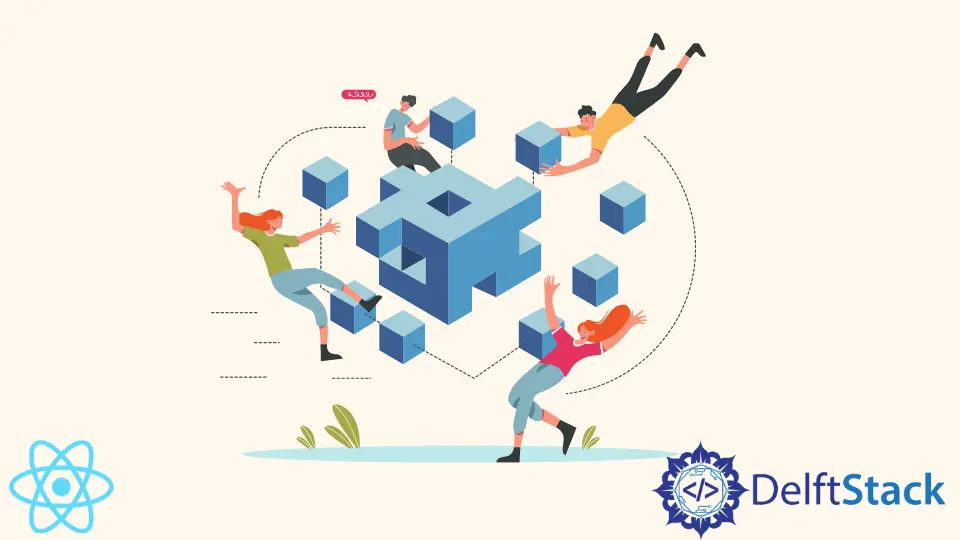
In React, we may need to sort and render an array of items. This post will look at how to sort and render an array of objects in React.
React Sort an Array
Because the Array.sort
function modifies the original array, we used the spread syntax (...
) to make a shallow duplicate of it before running sort()
.
Use the Array.map
function to draw the sorted array.
import React from 'react';
export default function App() {
const students = [
{id: 9, name: 'Shiv', country: 'Nepal'},
{id: 7, name: 'Kumar', country: 'Japan'},
{id: 5, name: 'Yadav', country: 'USA'},
];
return (
<div>
{[...students]
.sort((a, b) => a.id - b.id)
.map((student) => {
return (
<div key = {student.id}><h2>ID: {student.id} <
/h2>
<h2>Name: {student.name}</h2 >
<h2>Country: {student.country} < /h2>
<hr / >
</div>
);
})}
</div>);
}
Now, for what you’re presumably here for a bit more information on the .sort()
function. A callback function is sent as an argument to .sort()
.
The callback accepts two parameters that indicate two array entries to compare.
The return
statement within the function body comprises a ternary expression. It compares the first (first name) attribute of two array members: A and B.
Return 1 if element A has a greater first name than element B (e.g., A comes later in the alphabet); otherwise, return -1.
If the value 1 is returned, element A is sorted first. If the value -1 is returned, element B is sorted first.
Indeed, pressing the sort
button now sorts our entries from A to Z by the first name.
After sorting, we chained a call to the map()
function. If we need to render the sorted array, we may skip keeping it in an intermediate variable.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn