How to Set Cookies in React
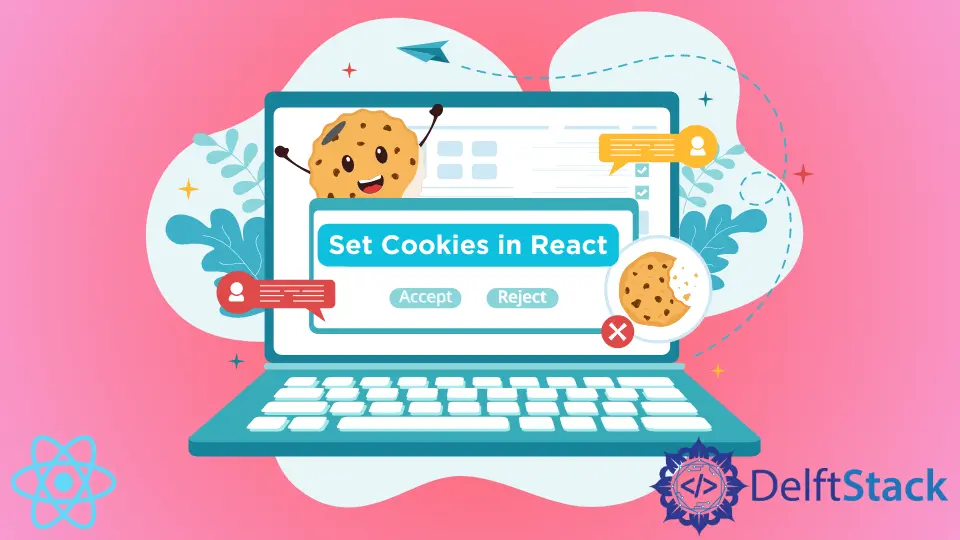
React developers aim to improve user experience by improving the efficiency and speed of the applications. Sometimes, cookies can be used to great effect and ensure a good experience for your users.
This article will demonstrate how to set cookies in React.
Cookies in React
Cookies are bits of HTTP code that can help you manage how to store bits of data on the server, so it will be automatically available the next time users visit your website.
For example, this information can be the username, email, user’s shopping cart, or browsing history. Cookies can help users resume their previous session seamlessly.
React is used to build single-page applications. The data is not loaded from the server, so we need to implement a custom approach to storing cookies in React.
Since we’re dealing with a JavaScript-based framework, we will use the document
web interface natively available in JavaScript.
Structure of Cookies in React
Cookies are usually structured as name-value
pairs. For example, this is a cookie to remember the user’s login until the next session:
document.cookie = `login=sample@gmail.com`
As you can see, we used the cookie
property of the document
interface and set it equal to the name-value
pair.
A cookie can include other details, such as when it should expire. If a parameter is not specified, a cookie will be deleted once the users have closed their browser.
If you intend to set multiple name-value
pairs of cookies in JavaScript, separate them with semicolons, like this:
document.cookie = `name=value; expires=expirationTime; path=domainPath`
Set Cookies in React
Let’s look at a practical example. Here, we have a simple App component in React.
export default function App() {
const handleClick = () => {
document.cookie = "username=admin";
console.log(document.cookie);
};
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
<button onClick={() => handleClick()}>Set cookies</button>
</div>
);
}
In this example, we set cookies in the handleClick()
method, set off by clicking a button. Alternatively, you can use lifecycle methods or the useEffect
hook to set up cookies when the component mounts, unmounts, or every time it updates.
You can try setting the cookies yourself in this live demo. Note that you have to open the preview in a new tab; otherwise, you won’t be able to see the updated cookies in the console.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn