How to Set Background Color With Inline Styles in React
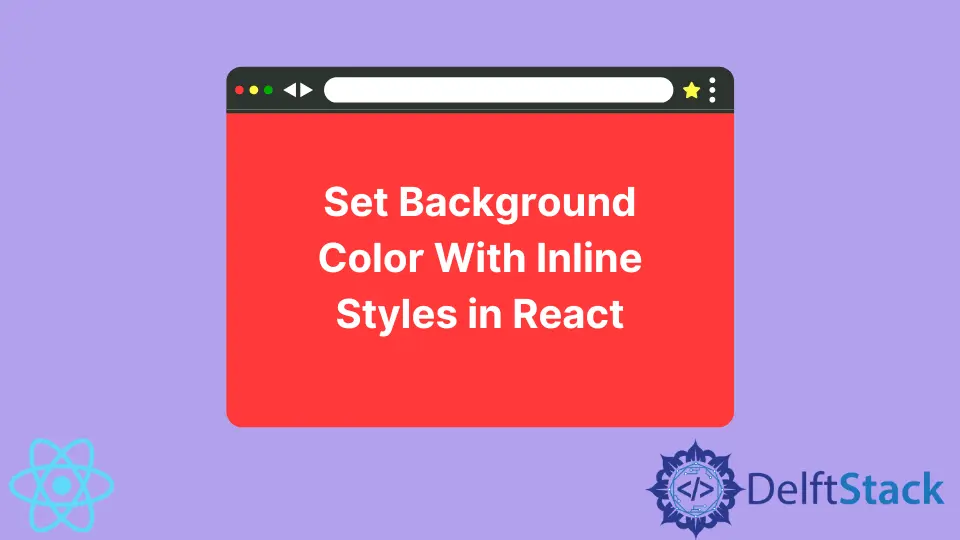
The ability to dynamically apply styles and classes is one of the best features of React. This article will discuss how to set inline styles to change background color in React.
Inline Styles in React
Inline styles allow you to style elements directly within JSX, a templating language of React. It helps you to easily structure your React application.
You can apply inline styles in React, just like in HTML. However, there are some differences between inline styles and CSS styles.
JSX looks a lot like HTML, but it is syntax sugar for writing JavaScript. For this reason, all CSS rules must be formatted as a JavaScript object.
Individual styles need to be written as key-value pairs in objects. CSS properties that a dash would separate are pushed together.
For example, the font-size
CSS property becomes fontSize
, and so on. Values of these properties need to be strings, integers, or Booleans.
Set Background Color With Inline Style in React
According to the rules we mentioned, CSS property background-color
becomes backgroundColor
in React inline styles. The value of this property needs to be a string, either a named color.
Syntax:
<div style={{ backgroundColor: "red"}}></div>
Alternatively, you can use HEX codes or RGBA, just like you would in CSS.
<div style={{ backgroundColor: "#ff0000"}}></div>
Let’s have an example of how to apply inline styles in React.
Code Snippet:
import "./styles.css";
export default function App() {
return <div style={{ backgroundColor: "red", padding: 100 }}></div>;
}
Output:
You can go to CodeSandbox for a live demo. The React inline styles are translated into normal CSS if you inspect the element.
Conclusion
Many beginners get confused with the syntax of inline styles in React. The important thing is to remember that CSS properties with multiple word names are combined and CamelCase.
Also, property names don’t need to be strings. Finally, the values must be either a string, an integer, or a Boolean.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn