How to Store Data in Session Storage in React
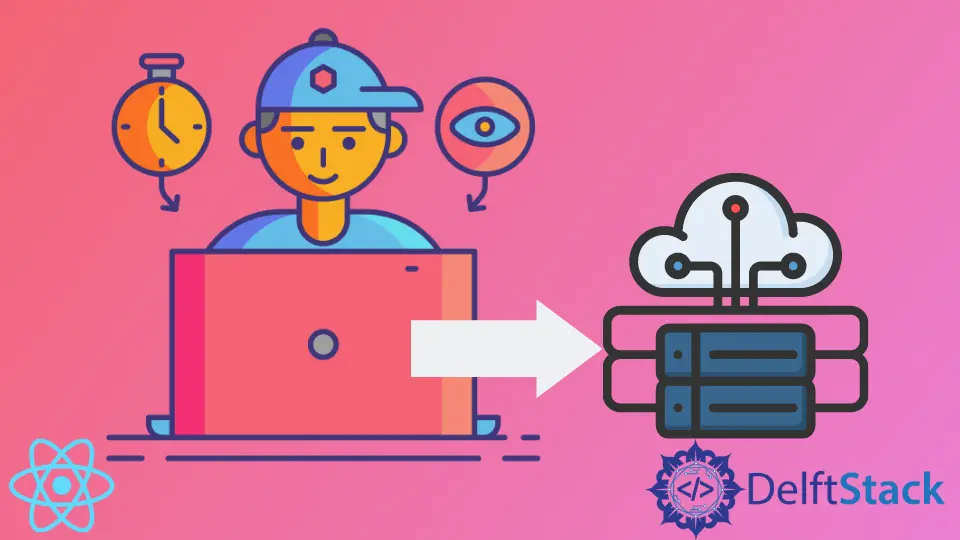
We will introduce how to store data in session
and display that data in React.
Session Storage in React
When building an application with multiple users and user roles, we need to use sessions and store some important data to perform queries based on user or user roles. Storing data in a session is the same as storing data in a local variable.
Let’s have an example in which we will store a user’s username on a button click using useState
. First, we will import useState
in App.js
.
# react
import React, { useState } from "react";
Next, we will define constants name
and setName
using useState
.
# react
const [name, setName] = useState("");
Now, let’s create a form in React, which will ask for the user’s name input and a submit button.
# react
<div className="App">
<h3>Session Storage in React</h3>
<form onSubmit={SessionDataStorage}>
<input
type="text"
placeholder="Enter your name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<button type="submit">submit</button>
</form>
</div>
As you notice in the code above, a function SessionDataStorage
will be called when the form is submitted. This function will store data in the session. So, let’s create that function now.
# react
const SessionDataStorage = (e) => {
e.preventDefault();
sessionStorage.setItem("name", name);
console.log(name);
};
So our app is complete now; let’s check how it looks on the frontend and how it works.
Output:
We successfully stored data in the session. Once the browser is closed, the data from the session is deleted.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn