How to Set Default Value of Select Element in React
-
Use the
defaultValue
Attribute to Set Default Value of Select Element in React -
Use
react-select
Library to Set Default Value of Select Element in React
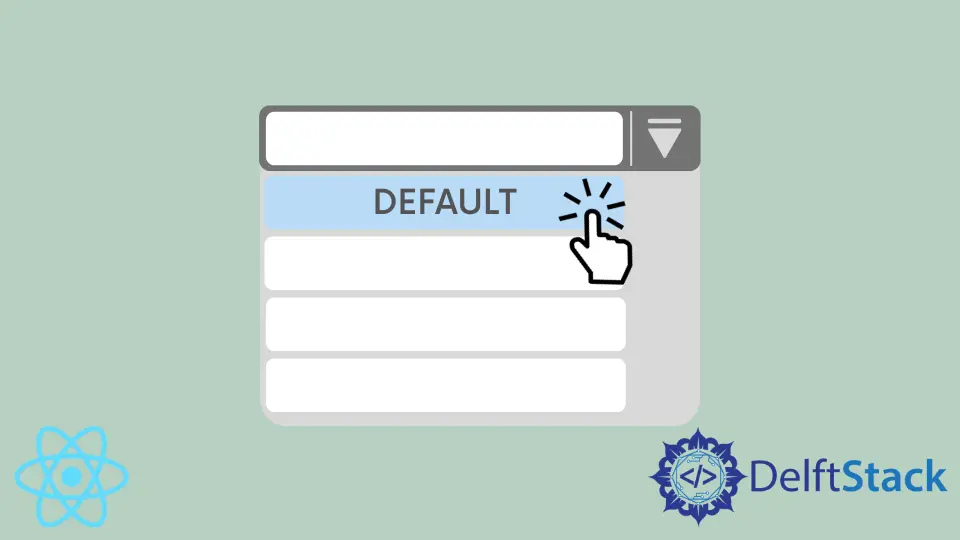
This article will discuss the <select>
and <option>
elements in React. We will explore how to access the selected values and set the default value.
Use the defaultValue
Attribute to Set Default Value of Select Element in React
React is a JavaScript based-UI library used to build powerful web applications. Any dynamic web application needs to accept user inputs and update the page accordingly.
The state
is one of the core React features, often used to store values from user inputs and trigger changes when necessary.
Unlike HTML, where the user’s choice is specified in the selected
attribute, React uses the value
attribute instead. This is done to keep all form and input elements consistent.
All controlled input components in React maintain the user’s selected value in the state
. The <select>
element, if it’s controlled, must have a value
attribute set to the selected value in the state.
To set the default value of the <select>
element, you can use the defaultValue
attribute in React. Let’s look at a sample code.
export default function Select() {
const [choice, setChoice] = useState();
return (
<div>
<select
value={choice}
defaultValue={"default"}
onChange={(e) => setChoice(e.target.value)}
>
<option value={"default"} disabled>
Choose an option
</option>
<option value={"one"}>One</option>
<option value={"two"}>Two</option>
<option value={"three"}>Three</option>
</select>
<h1>You selected {choice}</h1>
</div>
);
}
This controlled component sets the default value using the appropriately named defaultValue
attribute on the <select>
element.
Please note that the value of the defaultValue
attribute should be the same as the value
attribute of one of the options.
We use the onChange
handler to update the value of our state variable. You can check the live demo on CodeSandbox.
If your <select>
element is not controlled, you can go without setting the value
attribute on the select
element and each of the options
. You can use the onChange
event handler to update the state.
Use react-select
Library to Set Default Value of Select Element in React
The react-select
is a library that provides a custom Select
component and gives you the option to customize its look, functionality, and more.
To customize the options, you must provide an array of objects with key-value pairs; for instance, the options from our previous example are below.
import Select from 'react-select';
export default function select(){
let options = [{label: "One", value: "one"}, {label: "Two", value: "two"}, {label: "Three", value: "three"}]
return (
<Select options={options}
defaultValue={{label: "Choose one", value: ""}}></Select>
)
}
As you can see, the react-select
allows us to set the default value. There can only be a single default value, so the value of the defaultValue
attribute should be a single object with value
and label
properties.
All event handling and rendering within the Select
component imported from the react-select
package happens under the hood.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn