React Router vs React Router DOM
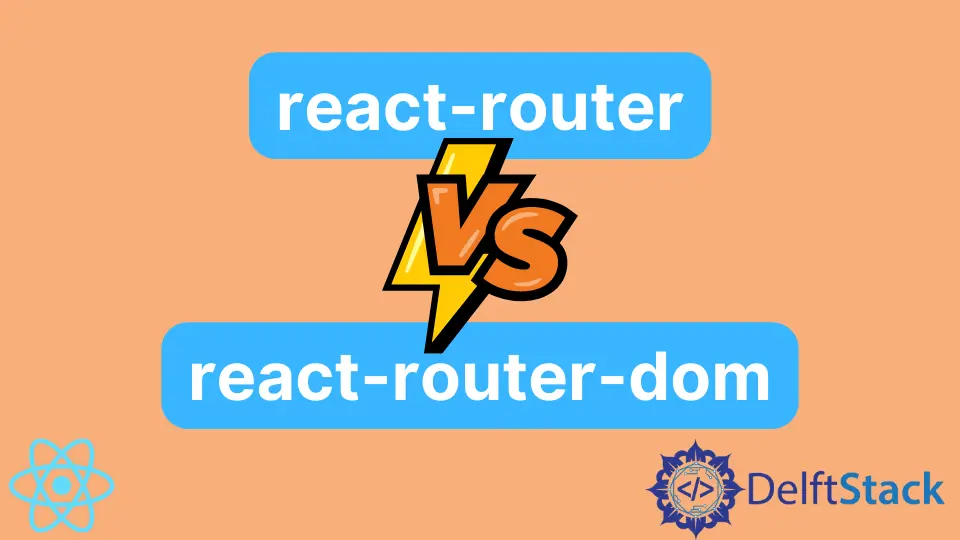
React is a library for building dynamic, visually appealing UIs with reusable components. It isn’t a full-fledged framework that includes routing and other advanced features.
If you’re building an advanced application in React, you will need a proper system to navigate between pages. You’ll have to install a separate library called React Router
to add this functionality to your React app.
React developers installing the React Router
library are often confused and don’t know to install the react-router
package or react-router-dom
. The two are similar in a lot of ways, but also different.
This article will compare them to help you figure out which one you need.
React Router vs. React Router DOM in React
React developers have three seemingly identical libraries to choose from: react-router
, react-router-dom
, and react-router-native
. Let’s start with the first one.
The react-router
library includes all the features and custom components from react-router-dom
and react-router-native
libraries. You can think of it as a broad library that unites two more specific libraries.
The react-router-native
is built for React Native, a framework for building mobile applications in React.
On the other hand, react-router-dom
is the library you need to add routing functionality to the web apps built in React. If you’re building a React application for browsers, then react-router-dom
is what you’re going to need.
In addition to all the custom components of the main react-router
library, react-router-dom
also includes the following components: <BrowserRouter>
, an improved version of the <Link>
component, and a <NavLink>
custom component, which you can use to set the styles for active
navigation items automatically.
You never have to import the main react-router
library in most cases.
Let’s import the custom Link
component from the react-router-dom
package and log it to the console.
import { Link } from "react-router-dom";
export default function App() {
console.log(Link);
return <div className="App">Sample app</div>;
}
Here’s what the Link
component looks like in the console.
You can see it on codesandbox.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn