How to Add the Regex to Path in React Router
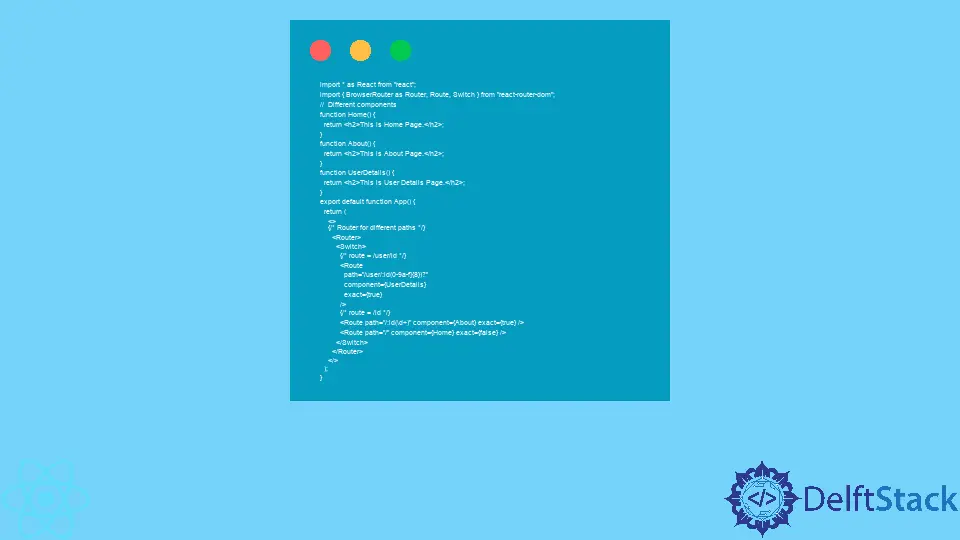
In this article, we will learn to add the regex to the react-router
paths. While creating the paths with the id or string requires validating the string from which we get the request.
The regular expressions allow us to validate the strings.
Use the react-router
V4 to Add Regex to the Path
In React.js, we can create the different routes using the react-router
library. For every route, we need to specify the paths, and we can add the regular expression in the path if the parameters we get in the path need to be validated.
In the example below, we have created three different routes. In the first and second routes, we added the regular expression to the path.
Also, we passed the exact={false}
as a prop to disable the exact match for the Home
component. So, when any route doesn’t match, we can redirect users to the Home
route.
The first route will accept the string containing the numbers and letters, and the second will accept only numbers. If routes do not match perfectly, it will show the home page.
Example Code:
import * as React from "react";
import { BrowserRouter as Router, Route, Switch } from "react-router-dom";
// Different components
function Home() {
return <h2>This is Home Page.</h2>;
}
function About() {
return <h2>This is About Page.</h2>;
}
function UserDetails() {
return <h2>This is User Details Page.</h2>;
}
export default function App() {
return (
<>
{/* Router for different paths */}
<Router>
<Switch>
{/* route = /user/id */}
<Route
path="/user/:id(0-9a-f]{8})?"
component={UserDetails}
exact={true}
/>
{/* route = /id */}
<Route path="/:id(\d+)" component={About} exact={true} />
<Route path="/" component={Home} exact={false} />
</Switch>
</Router>
</>
);
}
Output:
When we go to the path like user/234edf43
, it will show the UserDetails
component.
If we go to the path like /1234
, it will show the About
component.
The path like /abcd12
will show the Home
component as any other route is not matching.
Also, users can change regular expressions according to requirements, and if the user finds that the regular expression is not working correctly, add the question mark at the end of the regular expression, as we have added in the first route.