How to Render Component via onClick Event Handler in React
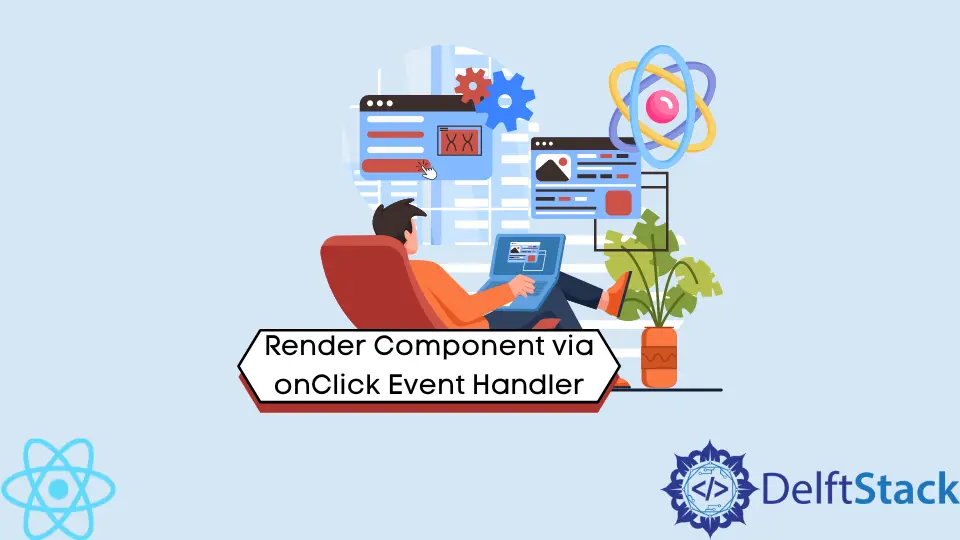
In this article, we will see how to set up React components to dynamically render components whenever the user clicks a button.
Render Component via onClick
Event Handler in React
When building web applications in React, you might want to conditionally render components based on the user’s input. This is possible by storing the user’s input in a state variable.
Functional React components return JSX code, which allows you to render (or not render) components based on the value of state variables.
To conditionally render components, you can use these two features and the JavaScript ternary operator.
Code:
export default function App() {
const [showComponent, setShowComponent] = useState(true);
return (
<div className="App">
{showComponent ? <Box /> : ""}
<button onClick={() => setShowComponent(!showComponent)}>
Hide and show Box
</button>
</div>
);
}
Output:
In the snippet above, we used the useState()
hook to initialize a state variable showComponent
to true
. We also create another variable, setShowComponent
, which will store a reference to the function that updates the state variable.
In JSX, we wrote a JavaScript expression, which evaluates the showComponent
variable and renders the <Box />
component if it is true. If not, it will render an empty string.
To write JavaScript expressions within JSX, you must wrap them with curly braces({}
).
We set the onClick()
event handler to update the value of the showComponent
state variable whenever someone clicks a button. We set the value of the state variable to the opposite of the current value.
If at the moment it’s true
, the box is rendered. Clicking the button sets it to false
, and the box is no longer rendered.
Notice how the position of the <Button>
DOM element changes. This is because, based on the value of the showComponent
variable, the <Box>
component (which is a div with fixed height and width) is rendered (or not rendered).
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn