How to Redirect to External URL in React
- Using the Window Location Object
- Using React Router’s Redirect Component
- Using the useEffect Hook for Automatic Redirects
- Handling External Redirects with Links
- Conclusion
- FAQ
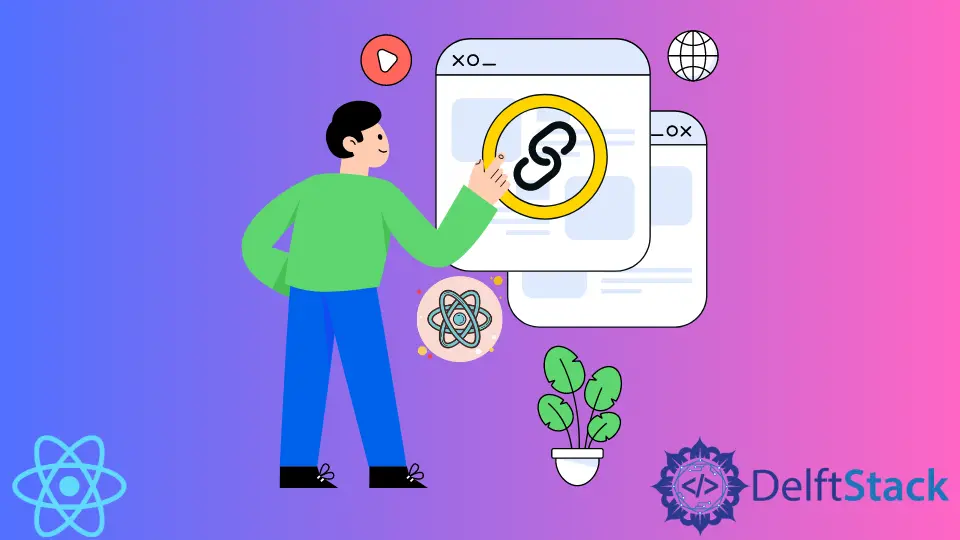
React is a powerful library for building user interfaces, and sometimes you may need to redirect users to an external URL. Whether you’re linking to a partner site, a documentation page, or an external service, knowing how to handle redirects efficiently is crucial.
In this tutorial, we will explore different methods to redirect to an external URL in React. We’ll cover various approaches, ensuring you have the tools you need to implement this functionality seamlessly in your applications. By the end of this article, you will be equipped with practical examples and a better understanding of how to manage external redirects in your React projects.
Using the Window Location Object
One of the simplest ways to redirect to an external URL in React is by using the window.location
object. This method allows you to change the current URL programmatically, which is particularly useful in event handlers or lifecycle methods.
Here’s how you can do it:
const redirectToExternalUrl = () => {
window.location.href = 'https://www.example.com';
};
// Usage in a component
const MyComponent = () => {
return (
<button onClick={redirectToExternalUrl}>
Go to External Site
</button>
);
};
In this example, we define a function called redirectToExternalUrl
, which sets window.location.href
to the desired external URL. When the button is clicked, the user is redirected to “https://www.example.com”. This method is straightforward and works well for most use cases. However, it’s important to note that this approach causes a full page reload, which might not be ideal for a smooth user experience in single-page applications.
Output:
User is redirected to https://www.example.com
Using React Router’s Redirect Component
If you are using React Router in your application, you might want to leverage its capabilities for redirection. While React Router primarily focuses on internal routing, you can still use it to redirect to external URLs with a little workaround.
Here’s how to do it:
import { Redirect } from 'react-router-dom';
const RedirectToExternal = () => {
return <Redirect to="https://www.example.com" />;
};
// Usage in a component
const MyComponent = () => {
return (
<div>
<h1>Redirecting...</h1>
<RedirectToExternal />
</div>
);
};
In this snippet, we create a RedirectToExternal
component that utilizes the Redirect
component from React Router. However, it’s important to remember that the Redirect
component is primarily designed for internal routes. To redirect to an external URL, you may need to handle this in a more controlled manner, such as through an onClick
event or by using useEffect
.
Output:
User is redirected to https://www.example.com
Using the useEffect Hook for Automatic Redirects
If you want to redirect users automatically after a certain action or condition, the useEffect
hook can be very handy. This approach is useful in scenarios like after form submissions or when a component mounts.
Here’s an example:
import React, { useEffect } from 'react';
const AutoRedirect = () => {
useEffect(() => {
window.location.href = 'https://www.example.com';
}, []);
return <div>Redirecting you to an external site...</div>;
};
// Usage in a component
const MyComponent = () => {
return <AutoRedirect />;
};
In this code, we use the useEffect
hook to execute the redirection logic when the component mounts. The window.location.href
is set to the external URL, and the user will be redirected automatically. This method is effective for scenarios where you want to control the timing of the redirection without user interaction.
Output:
User is redirected to https://www.example.com
Handling External Redirects with Links
For a more traditional approach, you can use anchor tags (<a>
) to create links that redirect users to external URLs. This method is straightforward and user-friendly.
Here’s how to implement it:
const ExternalLink = () => {
return (
<a href="https://www.example.com" target="_blank" rel="noopener noreferrer">
Visit External Site
</a>
);
};
// Usage in a component
const MyComponent = () => {
return <ExternalLink />;
};
In this example, we create an ExternalLink
component that renders an anchor tag. The href
attribute is set to the external URL, and we include target="_blank"
to open the link in a new tab. The rel="noopener noreferrer"
attribute is added for security reasons, preventing potential security risks associated with opening links in a new tab. This method is user-friendly and maintains a seamless experience within your React application.
Output:
User clicks the link and is redirected to https://www.example.com in a new tab
Conclusion
Redirecting to an external URL in React can be achieved through various methods, each with its own advantages. Whether you prefer using the window.location
object for a straightforward approach, leveraging React Router for internal navigation, or utilizing the useEffect
hook for automatic redirects, you now have the tools to implement this functionality effectively. Additionally, using anchor tags for external links provides a user-friendly option that aligns with web standards. Choose the method that best suits your application’s needs, and ensure a smooth user experience.
FAQ
-
How do I redirect to an external URL in React?
You can use thewindow.location.href
method to redirect users to an external URL in React. -
Can I use React Router to redirect to an external URL?
While React Router is primarily for internal routing, you can use it with some workarounds, but it’s generally better to usewindow.location
for external URLs. -
What is the best method for automatic redirects in React?
TheuseEffect
hook is a great way to handle automatic redirects when a component mounts or after an action. -
Is it safe to use target="_blank" in anchor tags?
Yes, but it’s recommended to includerel="noopener noreferrer"
for security reasons when usingtarget="_blank"
. -
Can I redirect after a form submission in React?
Yes, you can use theuseEffect
hook or handle the redirection in the form submission handler to redirect users after they submit a form.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn