How to Set the Text Color in React Native
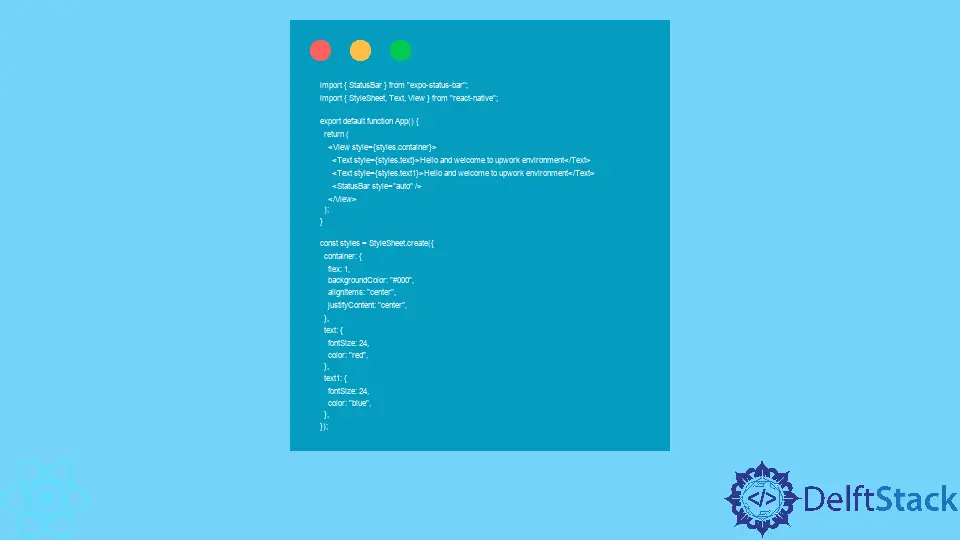
This article covers changing the text color in a Native React application. This example will be as straightforward as possible so that you may better grasp how to set the text content’s color in React Native.
Set the Text Color in React Native
To set the text color in your Text
component, you must utilize the color
attribute or property of the CSS design. Let’s see how to set text color in the React Native application.
import {StatusBar} from 'expo-status-bar';
import {StyleSheet, Text, View} from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello and welcome to upwork environment</Text>
<Text style={styles.text1}>Hello and welcome to upwork environment</Text>
<StatusBar style='auto' />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#000",
alignItems: "center",
justifyContent: "center",
},
text: {
fontSize: 24,
color: "red",
},
text1: {
fontSize: 24,
color: "blue",
},
});
In the above program, there are two Text
components. Each Text
component has its styles, and they get their styles from the StyleSheet
.
The first Text
component gets the style from text
which has its text color red
, whereas the second Text
component gets its style from text1
, which has its text color blue
.
Output:
In the above output, you can see two sentences of text with their color. You can change the color from the StyleSheet
component if you want to change it.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn