React Native Text Styles
- Method 1: Using the Text Component with Style
- Method 2: Combining Styles for Enhanced Effects
- Method 3: Using Custom Components for Advanced Styling
- Conclusion
- FAQ
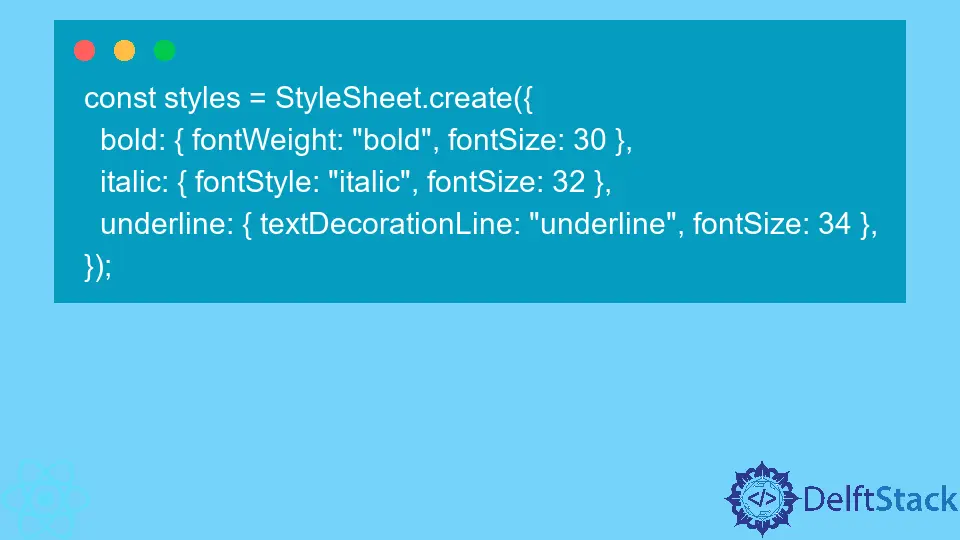
When developing applications using React Native, styling text can often be a crucial part of the user interface. One common requirement is to underline text, which can help emphasize specific information or create a visual hierarchy within your app.
In this article, we’ll explore how to effectively underline text in React Native, providing you with clear examples and explanations. Whether you’re a beginner or an experienced developer, understanding text styles in React Native will enhance your app’s aesthetic appeal and usability. So, let’s dive into the various methods to underline text in React Native!
Method 1: Using the Text Component with Style
The simplest way to underline text in React Native is by utilizing the built-in Text
component with the appropriate style. You can achieve this by using the textDecorationLine
property within the style object. This property allows you to specify how you want the text to appear, including underlining it. Here’s a straightforward example:
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const UnderlineTextExample = () => {
return (
<View style={styles.container}>
<Text style={styles.underlinedText}>This text is underlined!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
underlinedText: {
textDecorationLine: 'underline',
fontSize: 20,
color: 'blue',
},
});
export default UnderlineTextExample;
Output:
This text is underlined!
In this code, we import the necessary components from React Native and create a functional component called UnderlineTextExample
. Inside this component, we use a View
to center the text on the screen. The Text
component is styled with textDecorationLine: 'underline'
, which is the key property that adds the underlining effect. The fontSize
and color
properties further enhance the text’s visibility.
Method 2: Combining Styles for Enhanced Effects
Sometimes, you might want to combine multiple styles to create more visually appealing text. For instance, you can underline text while also applying other styles like font weight or color. This method allows you to achieve a more customized look for your text. Here’s how you can do it:
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const CombinedStylesExample = () => {
return (
<View style={styles.container}>
<Text style={styles.combinedText}>Underlined and Bold Text!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
combinedText: {
textDecorationLine: 'underline',
fontWeight: 'bold',
fontSize: 24,
color: 'green',
},
});
export default CombinedStylesExample;
Output:
Underlined and Bold Text!
In this example, we create another functional component called CombinedStylesExample
. Here, the Text
component is styled not only with textDecorationLine: 'underline'
but also with fontWeight: 'bold'
. This combination makes the text stand out even more. The larger font size and a vibrant color enhance its visibility, making it an excellent choice for headings or important information.
Method 3: Using Custom Components for Advanced Styling
If you need more control over your text styles, consider creating a custom text component that can handle various styles, including underlining. This approach is especially useful when you want to reuse the same styled text throughout your application. Here’s how you can create a reusable component:
import React from 'react';
import { Text, StyleSheet } from 'react-native';
const CustomText = ({ children, underlined }) => {
return (
<Text style={[styles.text, underlined && styles.underlined]}>
{children}
</Text>
);
};
const styles = StyleSheet.create({
text: {
fontSize: 18,
color: 'purple',
},
underlined: {
textDecorationLine: 'underline',
},
});
export default CustomText;
Output:
Your underlined text here!
In this code snippet, we define a CustomText
component that takes children
and an underlined
prop. If the underlined
prop is true, it applies the underlined
style, which includes the textDecorationLine: 'underline'
property. This modular approach allows you to easily create underlined text wherever you need it by simply using the CustomText
component with the appropriate prop.
Conclusion
Understanding how to underline text in React Native is essential for creating visually appealing and user-friendly applications. Whether you’re using the built-in Text
component, combining styles for enhanced effects, or creating a custom component for reusable styles, React Native provides the flexibility you need. By following the methods outlined in this article, you can ensure that your text not only conveys the right message but also looks great on any device. Happy coding!
FAQ
-
How do I underline text in React Native?
You can underline text in React Native by using thetextDecorationLine
property in theText
component’s style. -
Can I combine underlining with other text styles?
Yes, you can combine underlining with other styles like font weight and color to create custom text appearances. -
What is the best way to create reusable text components in React Native?
You can create a custom text component that accepts props to apply various styles, including underlining, making it reusable throughout your app. -
Is it possible to animate the underlining effect?
While React Native does not provide a built-in way to animate text decoration, you can achieve this effect using libraries like React Native Reanimated or by manipulating styles dynamically. -
Can I underline only part of the text in a single Text component?
Yes, you can achieve this by nesting multipleText
components within a parentText
component, applying the underline style to only the specific part you want to emphasize.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn