How to Align Text in React-Native
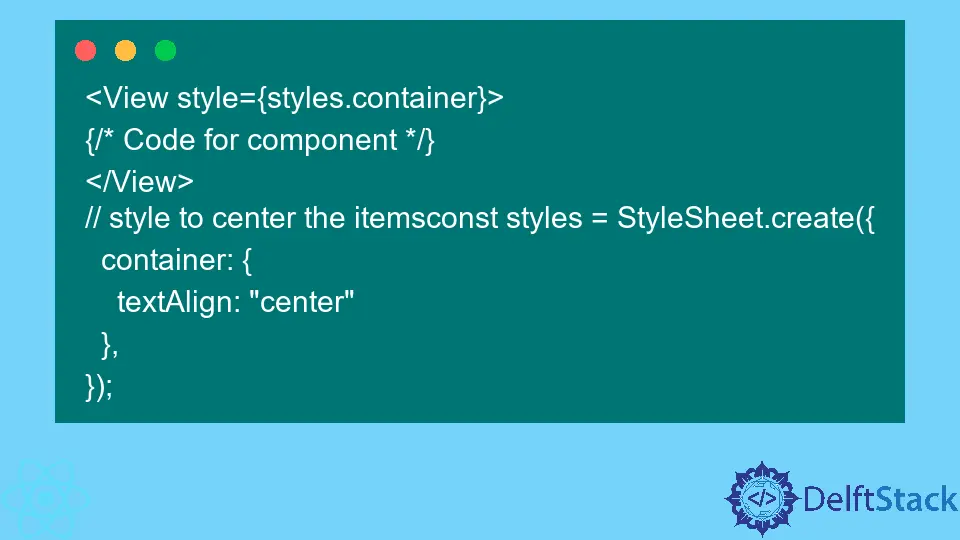
In this article, we will learn how to align texts at various positions, such as left, right, and center, in React-native. While developing the mobile application, there are many times developers require to align items to make an attractive UI.
Align Texts to the Center in React-Native
To align the texts in React-native, we need simple CSS. When we add textAlign: center
to any component, it will align items to the center.
Users can follow the syntax below to add textAlign: center
to any component.
<View style={styles.container}>
{/* Code for component */}
</View>
// style to center the itemsconst styles = StyleSheet.create({
container: {
textAlign: "center"
},
});
In the example below, we have created some Text
components and added them inside the single View
component. We have applied the container
style on the View
component.
In the container
style, we have added the textAlign: center
CSS property to center the content of the View
component, which users can see in the output.
Example Code:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={styles.container}>
<Text>Welcome to DelftStack!</Text>
<Text>Welcome to DelftStack!</Text>
<Text>Welcome to DelftStack!</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
width: "100%",
backgroundColor: "#fff",
textAlign: "center"
},
});
Output:
Align Texts to the Right in React-Native
The simplest way to align the texts to the right in React-native is to use the CSS property textAlign: Right
. Also, we will discuss some other ways to align text to the right.
In the below example, we’ve used the textAlign: right
CSS property for the Text
component and users can see in the output that the text is aligned to the right.
Example Code:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View>
<Text>Normal Postion of the text.</Text>
<Text style={styles.text}>Text is at Right</Text>
</View>
);
}
const styles = StyleSheet.create({
text: {
textAlign: "right"
},
});
Output:
Also, users can use the alignSelf
CSS property to align the text right. When we use the value flex-end
as the value of the alignSelf
CSS property, it aligns the text to the right.
Users can see the syntax below to use the alignSelf
CSS property.
<View>
<Text style={{alignSelf: 'flex-end'}}>This is a Demo Text!</Text>
</View>
Now, we will learn to align some text to the right and some to the left of a single row.
In the below example, we’ve created the View
component and added the flexDirection: row
as a CSS property to align their child component in a single row. In the child components, we have added the textAlign
CSS property according to requirements, and that’s how we can align some text to the right and some to the left in a single row.
Example Code:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={{flex: 1, flexDirection: 'row'}}>
{/* align left*/}
<View style={{flex: 1}}>
<Text>Left Text</Text>
</View>
{/* align right*/}
<View>
<Text style={{textAlign: 'right'}}>Right Text</Text>
</View>
</View>
);
}
Output:
In general, we don’t need to align a text to the left as it is used by default. Still, if users want to align the text to the left, they can use the textAlign: left
CSS property in React Native.
We learned to align text to the left and right in this article. Also, we learned to align the text of a single component or single row, which is very useful when a developer wants to put the text followed by the image in a single row.
Related Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop