React Native setTimeout Method
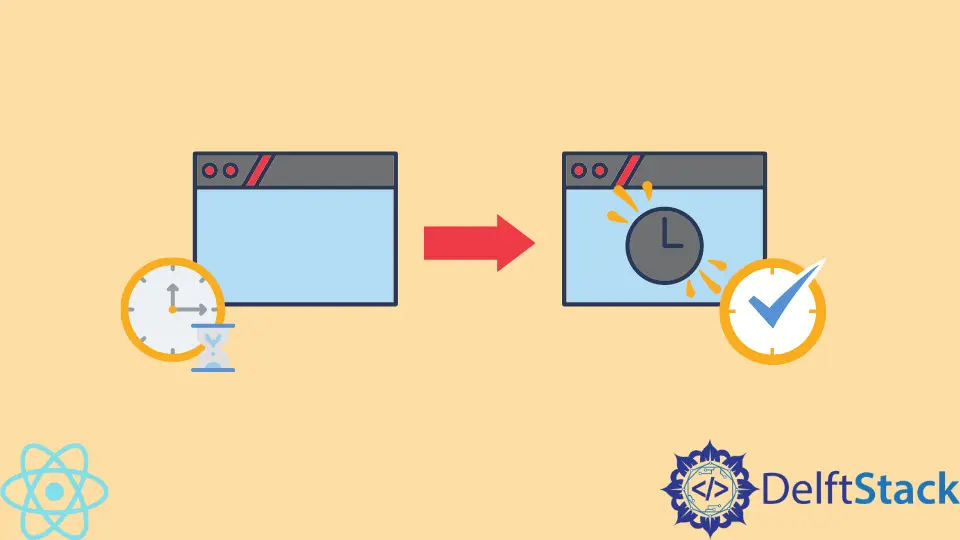
We will demonstrate in this short article how to implement setTimeout
in React Native.
Use the setTimeout
Method in React Native
We may occasionally need to execute code after a few seconds. In such circumstances, we use React Native’s JavaScript API setTimeout
.
The setTimeout
method performs a function after a specified time has passed.
Code Example:
import React from 'react';
import {Button, Image, StyleSheet, View} from 'react-native';
const App = () => {
const [image, setImage] = React.useState(null);
const showImage = () => {
setTimeout(() => {
setImage(
'https://www.casio.com/content/dam/casio/product-info/locales/us/en/timepiece/product/watch/G/GM/gm2/gm-2100-1a/assets/GM-2100-1A.png.transform/main-visual-pc/image.png');
}, 3000);
};
return (
<View style={styles.container}>
<View style={styles.imageContainer}>
<Image
source={
{ uri: image }}
style={
{ width: '100%', height: 160 }}
resizeMode='contain'
/>
</View>
<View style={styles.buttonContainer}>
<Button
title="Click On the button to see the image"
onPress={() => showImage()}
/>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 0.85,
justifyContent: 'center',
paddingHorizontal: 10,
},
buttonContainer: {
marginTop: 10,
},
imageContainer: {
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Here, we have code to delay a load of images using the setTimeOut
method, which slows the images’ loading by 3000 milliseconds, precisely 3 seconds. We have declared a showImage
method that uses a setTimeOut
method.
The showImage
method is invoked in the button, which triggers using the onPress
method of JavaScript.
Output:
The image will appear after 3 seconds when you click on the button.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn