How to Add Fixed Footer Feature to React Native App
- Understanding Sticky Footers in React Native
- Method 1: Using React Native’s Built-in Components
- Method 2: Using React Navigation
- Conclusion
- FAQ
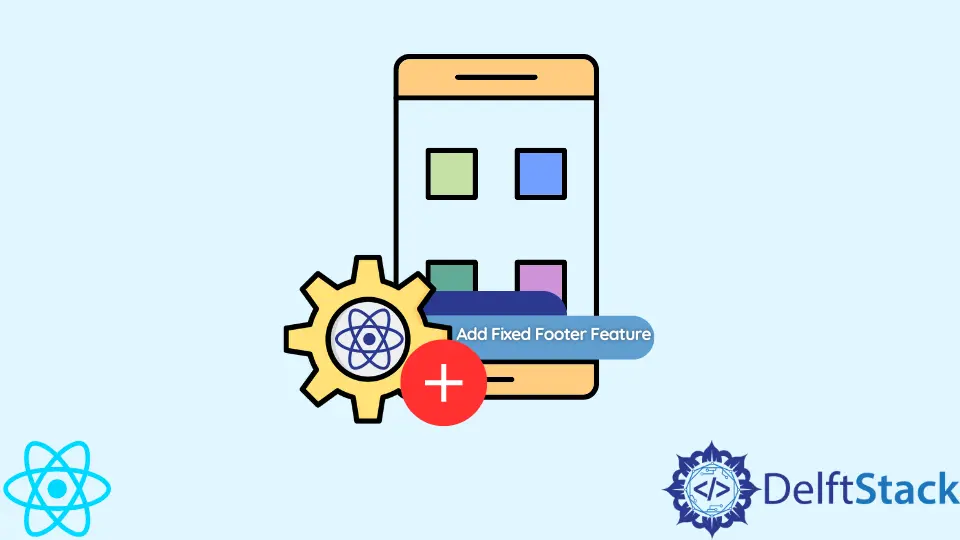
In the world of mobile app development, user experience is paramount. One effective way to enhance usability is by implementing a fixed footer in your React Native app. A sticky footer remains visible at the bottom of the screen, providing users with easy access to navigation or other essential features regardless of their position within the app.
In this article, we will explore the concept of sticky footers and guide you through the process of implementing this feature in your React Native application. Whether you’re a seasoned developer or a beginner, you’ll find the steps straightforward and easy to follow.
Understanding Sticky Footers in React Native
Before we dive into the implementation, let’s clarify what a sticky footer is. In simple terms, a sticky footer is a UI component that remains fixed at the bottom of the screen, regardless of the content above it. This feature is particularly useful in mobile applications, where screen real estate is limited, and users benefit from having essential navigation options readily available.
When designing your app, consider the following benefits of a sticky footer:
- Improved Navigation: Users can easily access key features without scrolling back to the top.
- Enhanced Usability: A fixed footer provides consistency across different screens, making your app easier to use.
- Increased Engagement: By keeping important actions visible, users are more likely to interact with them.
Now that we understand its significance, let’s move on to the methods for implementing a fixed footer in your React Native app.
Method 1: Using React Native’s Built-in Components
One of the simplest ways to create a fixed footer is by utilizing React Native’s built-in components. This approach involves using the View
component to create a footer that remains at the bottom of the screen.
Here’s a basic implementation:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<View style={styles.content}>
<Text>Main content goes here</Text>
</View>
<View style={styles.footer}>
<Text>Fixed Footer</Text>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
content: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
footer: {
height: 60,
backgroundColor: '#ccc',
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Output:
Main content goes here
Fixed Footer
In this code, we create a simple React Native app with a footer. The View
component serves as the main container, while the footer is defined at the bottom. By setting the flex
property to 1 for the content, we ensure that it takes up the available space above the footer. The styles are straightforward, with the footer having a fixed height and a background color for visibility.
This method is effective for basic applications. However, if you need more complex interactions or design, you might want to explore additional libraries or custom components.
Method 2: Using React Navigation
If your app relies on navigation, integrating a sticky footer with React Navigation can enhance your user experience significantly. React Navigation allows you to manage your app’s navigation state and provides a seamless way to implement a fixed footer.
Here’s how to set it up:
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
import { View, Text } from 'react-native';
const Tab = createBottomTabNavigator();
const HomeScreen = () => (
<View><Text>Home Screen</Text></View>
);
const SettingsScreen = () => (
<View><Text>Settings Screen</Text></View>
);
const App = () => {
return (
<NavigationContainer>
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeScreen} />
<Tab.Screen name="Settings" component={SettingsScreen} />
</Tab.Navigator>
</NavigationContainer>
);
};
export default App;
Output:
Home Screen
Settings Screen
In this example, we use createBottomTabNavigator
from React Navigation to create a tabbed interface. The Tab.Navigator
component automatically generates a footer that remains fixed at the bottom of the screen. Each tab represents a different screen, allowing users to switch between them seamlessly.
Using React Navigation for a sticky footer is a great choice for applications with multiple screens, as it not only provides a fixed footer but also manages navigation effectively. This method is particularly beneficial for apps that require a more complex structure.
Conclusion
Implementing a fixed footer in your React Native app can significantly enhance the user experience by providing easy navigation and consistent access to essential features. Whether you choose to use basic components or leverage React Navigation, the methods outlined in this article are straightforward and effective. By keeping your footer fixed at the bottom, you ensure that your users can easily interact with your app, leading to higher engagement and satisfaction. Start integrating sticky footers today and watch your app’s usability soar!
FAQ
-
What is a sticky footer in React Native?
A sticky footer is a UI component that remains fixed at the bottom of the screen, providing easy access to navigation or other essential features. -
How can I create a sticky footer in React Native?
You can create a sticky footer using React Native’s built-in components or by utilizing React Navigation for a more structured approach. -
Are there any libraries for implementing fixed footers in React Native?
Yes, libraries like React Navigation provide built-in support for creating fixed footers through tab navigators. -
Can I customize the appearance of the footer?
Absolutely! You can customize the styles of the footer using theStyleSheet
in React Native to match your app’s design. -
Is a sticky footer suitable for all types of apps?
While a sticky footer can enhance usability in many apps, it may not be necessary for simpler applications with minimal navigation needs.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn