How to Log to the Console in React Native
-
Use
console.log()
to Log to the Console in React Native - Use Chrome Developer Tools to Log to the Console in React Native
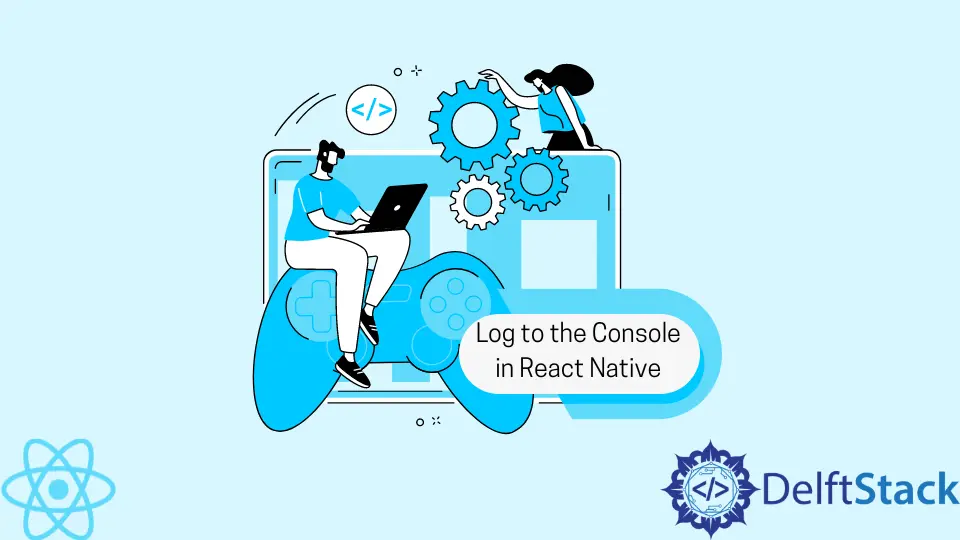
When developing any application, logging into the console is necessary for debugging. Its console is easy to access for web apps, and web developers can see the values logged to the console.
Let’s see how to do the same for mobile applications created with React Native.
Use console.log()
to Log to the Console in React Native
Logging can be an invaluable tool for debugging mobile applications. Still, many developers avoid it altogether because logging to the console in React Native is not as intuitive as it is in the browser.
React Native allows you to develop web applications with JavaScript. So developers can use the console.log()
method to log something in the terminal.
The problem is that console
in mobile applications is more difficult to access. Let’s look at a simple React Native component that calls the console.log()
method.
const SomeComponent = () => {
const variable = 20
console.log(variable)
return (
<View>
<Text>Hello, world!</Text>
</View>
)
}
In React Native, to access the current value logged to the console, we must run one of these commands.
react-native log-ios
react-native log-android
It’s probably clear, but the first command is for iOS, and the second is for Android.
Use Chrome Developer Tools to Log to the Console in React Native
Another great way to see logged values is to connect your application with Chrome Developer Tools and see the logged values there.
To do this, you must first install the browser. Then you must use the appropriate command to run the app, either for iOS or Android.
The next step is to open the developer
menu and choose the Debug JS Remotely
option. Selecting this option will open the Debugger
page in the browser.
Finally, you must navigate to the Developer Options
page (Tools -> More Tools -> Developer Options) and open the console
tab.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn