How to Include an Image in React Native
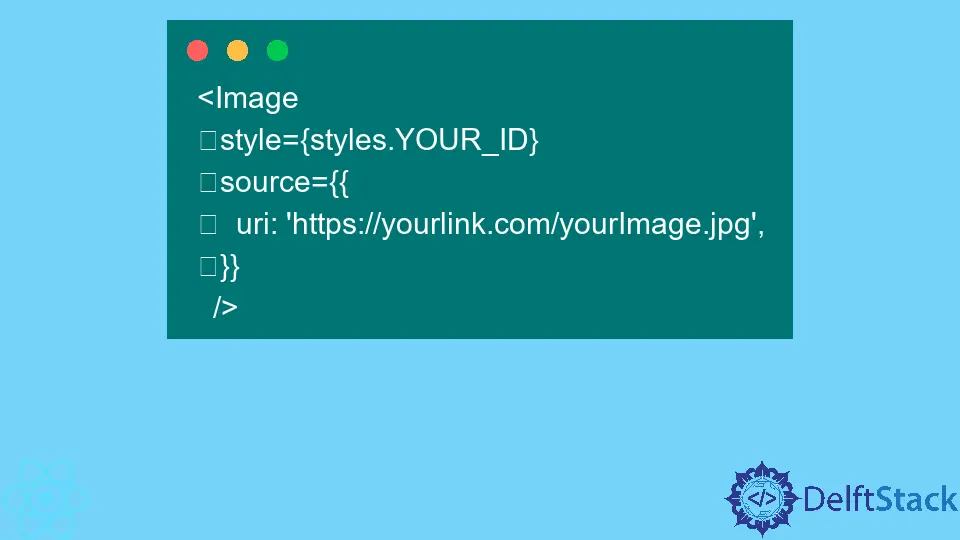
When designing an app, you must work with icons and logos.
These images can be in different formats. In React-Native, it is easy to include an image in your app.
This article will show how we can include images and size them on the React-Native app. Also, we will discuss the topic by following the necessary examples and explanations to make the topic easy.
To include an image on your React-Native app, follow the below steps:
-
Import the
Image
from thereact-native
package. -
Inside the
return()
, implement theimage
tag as follows:<Image style={styles.YOUR_ID} source={{ uri: 'https://yourlink.com/yourImage.jpg', }} />
-
Lastly, style (size) the image using the specific ID.
As we discuss the steps required to include an image on the React-Native
app, let’s see an example to make it clearer to us.
an Example of Including Image and Size on React-Native
App
In our below example, we illustrated how we could include images in our React-Native app. The code for our example is below.
// importing necessary packages
import {StatusBar} from 'expo-status-bar';
import {Image, StyleSheet, Text, View} from 'react-native';
export default function App() {
return ( // Including the image
<View style={styles.container}>
<Text>Simple Image</Text>
<Image
style={styles.img}
source={{
uri: 'https://st2.depositphotos.com/1031343/6062/v/450/depositphotos_60622807-stock-illustration-keep-it-simple-stamp.jpg',
}
}
/>
<StatusBar style="auto" / > <
/View>
);
}
const styles = StyleSheet.create({ / /
Providing style.container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
img: {
width: 100,
height: 100,
resizeMode: 'contain',
},
});
The purpose of all necessary lines regarding the steps shared above in the example is left as comments. Now when you run the app, you will get the below output on your screen.
Please note that the code shared above is created in React-Native
, and we used the Expo-CLI
to run the app.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop