How to Include Icon Button in React Native
- Understanding Icon Buttons
- Setting Up Your React Native Environment
- Installing React Native Vector Icons
- Creating an Icon Button
- Customizing the Icon Button
- Adding Functionality to the Icon Button
- Conclusion
- FAQ
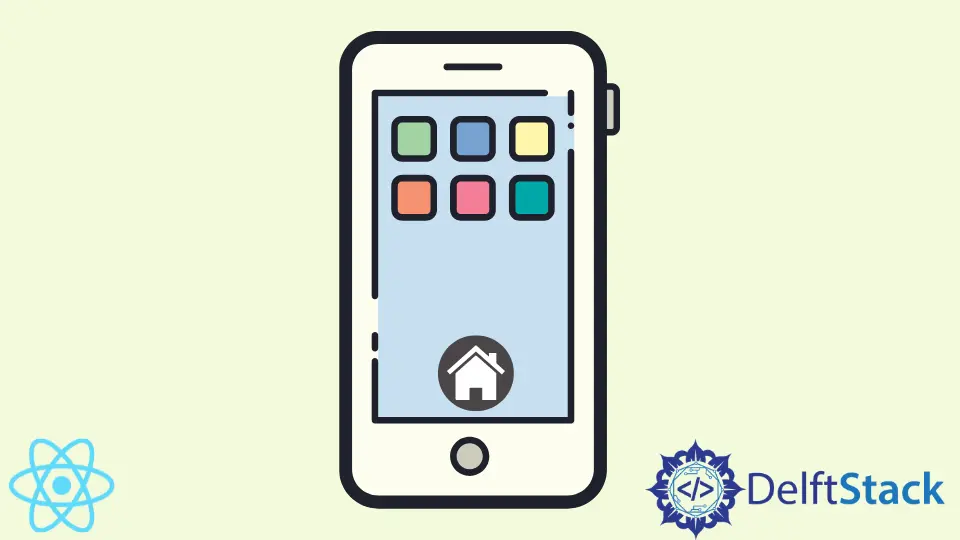
In the world of mobile app development, React Native has emerged as a popular framework for building cross-platform applications. One of the essential components that can enhance user experience is the icon button. Icon buttons serve as a visual cue that can make your app more intuitive and engaging.
In this tutorial, we will explore how to include an icon button in React Native. By the end, you’ll be able to create a stunning icon button that fits seamlessly into your app’s design. Whether you’re a beginner or an experienced developer, this guide will provide you with all the information you need to implement icon buttons effectively.
Understanding Icon Buttons
Before diving into the coding aspect, let’s clarify what an icon button is. An icon button is a clickable element that contains an icon, which can represent an action or a function. These buttons are not only visually appealing but also save screen space, especially in mobile applications where real estate is limited.
React Native provides a straightforward way to implement icon buttons using libraries like react-native-vector-icons
, which offers a wide array of customizable icons. In the following sections, we will walk through the steps to install this library and create an icon button.
Setting Up Your React Native Environment
To include an icon button in your React Native project, you first need to set up your environment. This involves ensuring that you have Node.js installed and that you have created a React Native project. If you haven’t done this yet, you can follow these steps:
-
Install Node.js from the official website.
-
Create a new React Native project using the command:
npx react-native init YourProjectName
-
Navigate to your project directory:
cd YourProjectName
Once your environment is set up, the next step is to install the necessary library for using icons.
Installing React Native Vector Icons
To add icon buttons to your app, you need to install the react-native-vector-icons
library. This library includes a collection of customizable icons that you can easily integrate into your project. Here’s how to install it:
Run the following command in your project directory:
npm install react-native-vector-icons
After installing, link the library to your project. For React Native versions 0.60 and above, auto-linking is supported, so you may not need to do anything further. However, if you encounter any issues, you can manually link it using:
react-native link react-native-vector-icons
Once the library is installed, you are ready to create your icon button.
Creating an Icon Button
Now that you have installed the necessary library, let’s create an icon button. Below is a simple example of how to implement an icon button using react-native-vector-icons
.
import React from 'react';
import { View, TouchableOpacity } from 'react-native';
import Icon from 'react-native-vector-icons/FontAwesome';
const App = () => {
return (
<View>
<TouchableOpacity>
<Icon name="home" size={30} color="#4F8EF7" />
</TouchableOpacity>
</View>
);
};
export default App;
In this code, we are importing TouchableOpacity
from React Native to create a clickable button. We also import Icon
from react-native-vector-icons/FontAwesome
, which allows us to use an icon from the FontAwesome collection. The name
prop specifies which icon to display, while size
and color
allow us to customize its appearance.
The TouchableOpacity
component is essential here as it provides feedback when the user presses the button. You can replace "home"
with any other icon name from the FontAwesome library to customize your button further.
Customizing the Icon Button
Customization is key to making your icon button fit your app’s design. You can change the size, color, and even add additional styles. Here’s an example of a more customized icon button:
import React from 'react';
import { View, TouchableOpacity, StyleSheet } from 'react-native';
import Icon from 'react-native-vector-icons/FontAwesome';
const App = () => {
return (
<View style={styles.container}>
<TouchableOpacity style={styles.button}>
<Icon name="cog" size={40} color="#FFFFFF" />
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#282C34',
},
button: {
backgroundColor: '#4F8EF7',
borderRadius: 20,
padding: 10,
},
});
export default App;
In this code, we added styles using StyleSheet.create
to enhance the button’s appearance. The button now has a background color, padding, and rounded corners, making it more visually appealing.
You can play around with the backgroundColor
, borderRadius
, and other styles to match your app’s theme perfectly.
Adding Functionality to the Icon Button
An icon button is only as good as its functionality. You can easily add actions to your button using the onPress
event. Let’s modify the previous example to include an alert when the button is pressed.
import React from 'react';
import { View, TouchableOpacity, StyleSheet, Alert } from 'react-native';
import Icon from 'react-native-vector-icons/FontAwesome';
const App = () => {
const showAlert = () => {
Alert.alert('Button Pressed!', 'You pressed the icon button.');
};
return (
<View style={styles.container}>
<TouchableOpacity style={styles.button} onPress={showAlert}>
<Icon name="bell" size={40} color="#FFFFFF" />
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#282C34',
},
button: {
backgroundColor: '#4F8EF7',
borderRadius: 20,
padding: 10,
},
});
export default App;
In this example, we defined a function called showAlert
, which triggers an alert when the button is pressed. This adds interactivity to your icon button, making it more than just a visual element.
Output:
An alert will pop up saying "Button Pressed!" when the icon button is clicked.
By incorporating functionality, you can create a more engaging user experience.
Conclusion
Including an icon button in your React Native application can significantly enhance user experience. With the help of libraries like react-native-vector-icons
, you can easily add customizable icons that serve functional purposes. In this tutorial, we covered how to set up your environment, install the necessary library, create an icon button, customize it, and even add functionality. By following these steps, you can make your app more intuitive and visually appealing.
Incorporating icon buttons is a great way to improve user interaction and create a polished look for your application.
FAQ
-
How do I install React Native Vector Icons?
You can install it using the command npm install react-native-vector-icons in your project directory. -
Can I customize the size and color of the icons?
Yes, you can customize the size and color by using the size and color props in the Icon component. -
What types of icons are available in React Native Vector Icons?
The library offers a variety of icons from different icon sets like FontAwesome, MaterialIcons, and more. -
How do I add functionality to my icon button?
You can add functionality by using the onPress event in the TouchableOpacity component. -
Is it necessary to link the library for React Native versions above 0.60?
No, auto-linking is supported in versions 0.60 and above, so linking is generally not required.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop