How to Format Currency in React Native
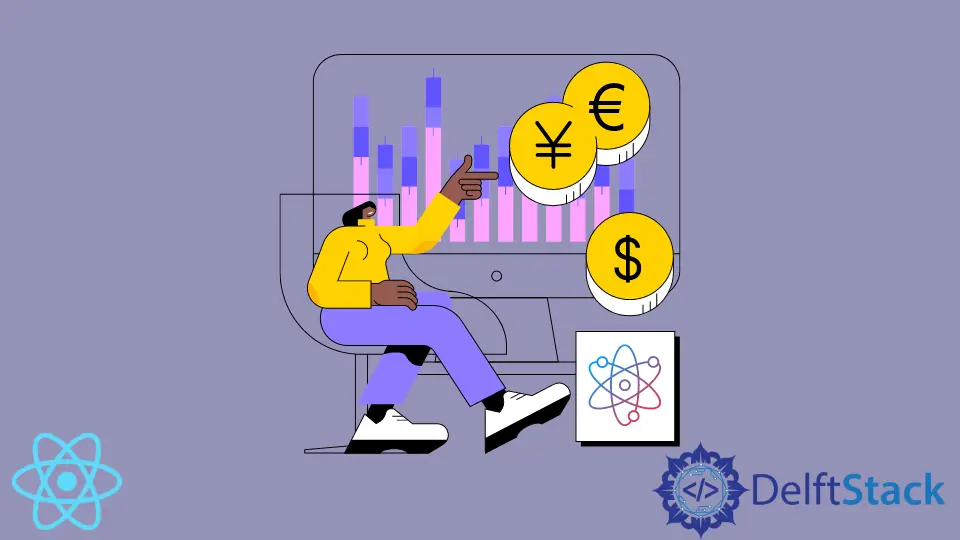
This tutorial will look at formatting a number to currency when using React Native. Using React Native, we can use the react-number-format
library to format a number to a currency.
React Native Format Currency
First, you must install this library to use the react-number
format. You can install this library using this command:
npm i react-number-format
After installing the library, we have to import NumberFormat
from react-number-format
in the React application.
import NumberFormat from 'react-number-format';
Then use it by writing the following code:
import * as React from 'react';
import {Text, View} from 'react-native';
import NumberFormat from 'react-number-format';
export default function App() {
return (
<View>
<NumberFormat
value = {12345} displayType = 'text'
thousandSeparator
prefix = '$'
renderText = {(value) => <Text>{value}</Text>}
/>
</View>
);
}
In the above code, we can see that there are different components in the primary function.
NumberFormat
consists of additional features to format a number in input or text. Some parts are value
, displayType
, thousandSeparator
, prefix
, etc.
value
is taken as the number to format. It might be a prepared text or a float number.
The isNumericString
prop should be proper if the value is a string representation of a number (unformatted).
displayType
specifies how the number formatted is rendered. If the input is given, an input element is generated where formatting is applied as characters are entered. If text
, it formats the supplied value in a span like standard text.
thousandSeparator
is used to separate numbers based on their place value, such as thousands. On the other hand, prefix
is added just before the number to generalize the currency symbol.
If you want to render formattedValue
in an element other than span, there is a renderText
function that might be helpful. Additionally, it gives back any custom props added to the component, allowing them to be passed on to the rendered element.
Output:
In the above program, we have just put the value as a number. In the output, we can see that the currency is in a pretty well format.
Hence, using React Native, we can use the react-number-format
library to format a number into a currency.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn