React Native Absolute Position
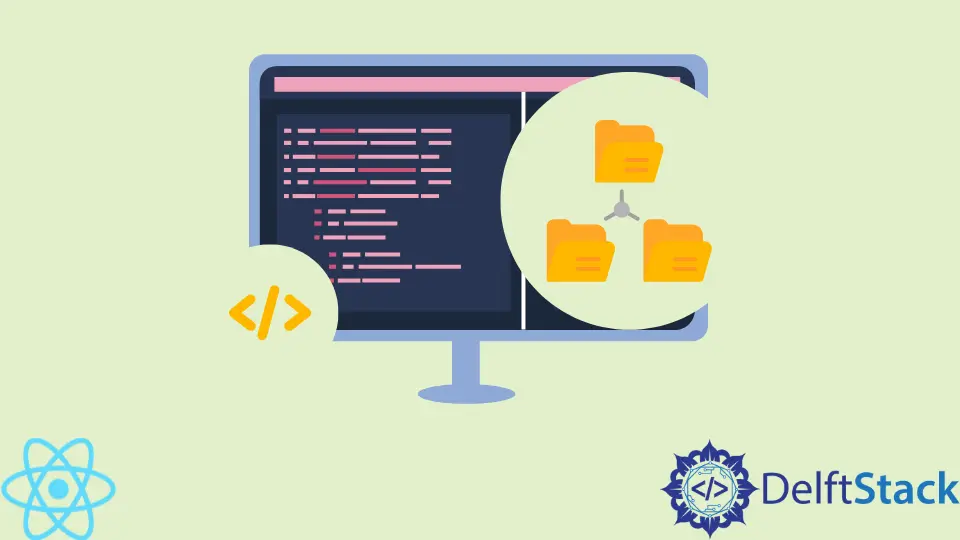
Although everything in React Native is set to a relative by default, absolute positioning is always related to the parent. Position in React Native is comparable to standard CSS.
React Native Absolute Position
Set the child to have an absolute position if you wish to place it relative to its parent by a certain amount of logical pixels.
Let’s see an example.
<>
<View
style={{
marginTop: 40,
height: 100,
backgroundColor: "#ccc",
}}
>
<View
style={{
height: 100,
width: 100,
backgroundColor: "red",
position: "absolute",
right: 0,
bottom: 0,
}}
/>
</View>
</>
The code above shows a main view
component; inside the main component, there is a child view
component.
If we change the parent component, then the child component remains inside the parent component.
Output:
Here in this screenshot, you can see two components, i.e., one having gray color, one having red color. The gray color component is the parent component, whereas the red component is the child component.
If we make changes in the parent component (like making height 100%, i.e., flex = 1), the child component will remain in the parent component.
<>
<View style={{
flex: 1,
marginTop: 40,
backgroundColor: '#ccc',
}}>
<View style={{
height: 100,
width: 100,
backgroundColor: 'red',
position: 'absolute',
right: 0,
bottom: 0,
}} />
</View>
</>
Output:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn