How to Add Logo in React
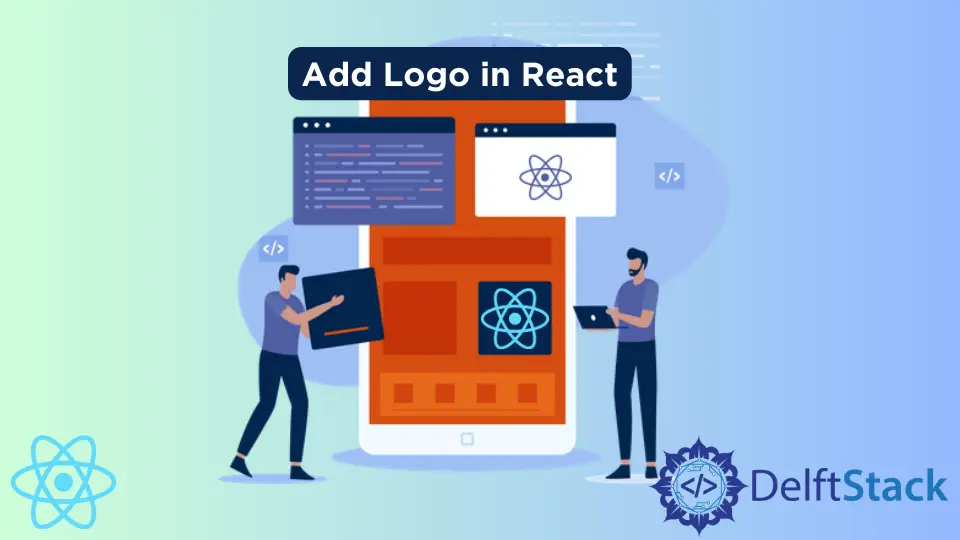
We will introduce how to add a logo
in React application.
Add Logo in React
Nowadays, every website has its logo and images.
When we build a React app, we first want to change the logo, and some people find it difficult to display the image in a React app. We will discuss displaying your logo in a React application with a simple code.
First of all, we will save our logo
in the src
folder. Once we have saved our logo, now we will import it inside the file we want to display it.
Use the following code to import the logo.
# react
import logo from './logo.svg';
Once we have imported our logo inside the page where we want to display it, we will create a view for it.
# react
<img src={logo} className="App-logo" alt="logo">
In the code provided, we can see that in the src
attribute, we are calling a variable {logo}
. This variable will output the URL
of the logo we imported.
We will write the CSS
code to resize the logo according to our needs.
# react
.App-logo{
width: 150px;
}
Let’s check our logo.
Output:
We have now learned how to easily display our images or logos in the React application.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn