How to Set the for Attribute of the Label Element in React
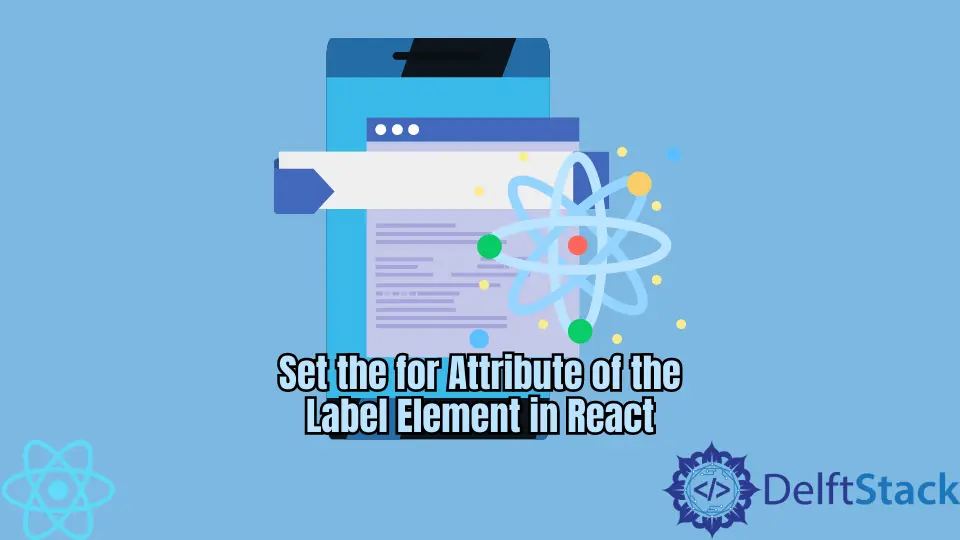
Some elements, such as the label element from HTML, are slightly different in React (JSX). This article will discuss the differences and show you how to set the for
attribute of label elements in React.
Set the for
Attribute of the Label Element in React
If you try to create a couple of <label>
and <input>
elements in JSX, you need to link them. That’s usually the purpose of the for
attribute on the <label>
element.
If you follow this pattern from HTML and set the for
attribute on this type of element, it will not work.
Tools that compile JSX into regular HTML will not include the for
attribute in the final HTML output.
In addition to that, the console will display warnings that urge you to use the htmlFor
attribute instead.
Properly Set the for
Attribute of the Label Element in React
To couple <label>
elements with specific inputs, you only have to remember that in JSX, we use htmlFor
instead of the for
attribute from HTML.
Let’s look at an actual example.
export default function App() {
return (
<div className="App">
<label htmlFor="nameField">Enter your name</label>
<input type="text" name="nameField"></input>
</div>
);
}
Two things to keep in mind: to associate it with a label element, you must set the name attribute on the <input>
element.
Then you can set the htmlFor
attribute of the text to the same value, and the text will be associated with the input.
View the live example on CodeSandbox. You can look into the source file to check the plain HTML version of our React application.
If you do, you’ll see that the <label>
element does have the for
attribute, as it should.
Change of Attributes From HTML to JSX
Some attributes from plain HTML are absent from React, others are renamed, and few can be found in React but not in HTML. For instance, the checked
attribute on checkboxes and radio buttons lets you quickly access their current value and control these components.
The class
attribute becomes className
because class
is a reserved word in React.
When setting attributes like htmlFor
or onChange
, consider that React is case sensitive, so don’t forget to use the camelCase convention.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn