How to Use the onChange Event in React
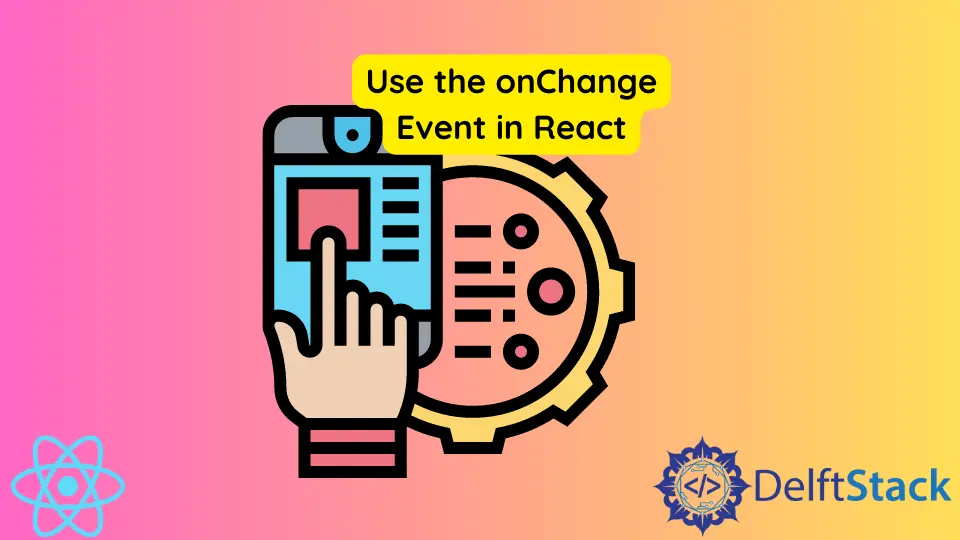
The event system is one of the most useful features included in React framework.
onChange
event, in particular, is one of the most frequently handled events in React. It is based on the well-known onchange
attribute in vanilla JavaScript, but the onChange
event in React includes a few additional features.
It is also written in camelCase to help developers differentiate it from regular JavaScript events.
Understanding how exactly the onChange
event works can help you write more powerful, dynamic forms.
onChange
With React Inputs
In React, the onChange
event occurs when the users’ input changes in any way. An input can change when the user enters additional text, selects a different option, unchecks the checkbox, or other similar instances.
Imagine a situation when you have a checkbox input and need to store users’ choice (a boolean
value) in the state. In this scenario, onChange
would be extremely useful. Any time the user changes their mind about input and unchecks it, the state will be updated.
Here’s an example:
return (
<input type="checkbox" onChange={(e) => this.setState("status": e.target.value)}/>
)
This is a simple demonstration of how useful an onChange
event can be.
We’re making this element a controlled input by storing the input value in our component state
. This term describes inputs whose internal state is merged with the state
of react component to provide a single source of truth.
Note that in JSX, the JavaScript expressions need to be placed between two curly braces. We could also define handler separately and invoke it within the curly braces.
For example:
<input type="checkbox" onChange={(e) => handleChange(e)}/>
For more complex handler functions, this would be a more readable approach.
Trigger onChange
Event in React Manually
In some cases, you might need to bypass the default behavior and trigger the onChange
event manually.
Imagine the browser auto-filled your password, so the onChange
doesn’t fire, but you need it to perform an animation. In this scenario, you have to trigger onChange
manually.
To avoid duplicate instances of the same events, sometimes React intentionally swallows some events and does not execute them. The solution is to use the native value setter setNativeValue()
. To get an in-depth understanding of the issue, check out this helpful blog post.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn