Image Tag in React
Rana Hasnain Khan
Dec 28, 2021
React
React Image
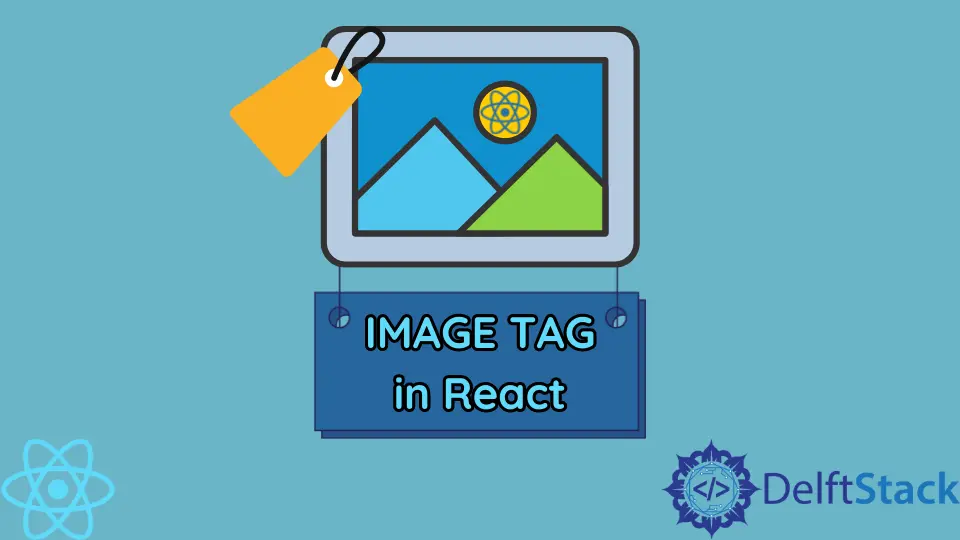
We will introduce how to add an image in React.
Image Tag in React
First, we will add a div
with class root
in the index.html
file.
# react
<div id="root"></div>
In the index.tsx
file, we will define the name
, url
, and title
of the image.
# react
import React, { Component } from 'react';
import { render } from 'react-dom';
import Hello from './Hello';
import './style.css';
interface AppProps { }
interface AppState {
name: string;
url: string;
title: string;
}
class App extends Component<AppProps, AppState> {
constructor(props) {
super(props);
this.state = {
name: 'React',
url: 'https://images.pexels.com/photos/8549835/pexels-photo-8549835.jpeg?auto=compress&cs=tinysrgb&dpr=2&h=650&w=940',
title: "Vase Image"
};
}
render() {
return ();
}
}
render(<App />, document.getElementById('root'));
We have taken an example picture. Now we will return an image by passing values in the image tag.
# react
<div>
<img src={this.state.url} alt={`${this.state.title}'s picture`} className="img-responsive" />
<span>Hello {this.state.name}</span>
</div>
So, our code in index.tsx
will look like the one below.
# react
import React, { Component } from 'react';
import { render } from 'react-dom';
import Hello from './Hello';
import './style.css';
interface AppProps { }
interface AppState {
name: string;
url: string;
title: string;
}
class App extends Component<AppProps, AppState> {
constructor(props) {
super(props);
this.state = {
name: 'React',
url: 'https://images.pexels.com/photos/8549835/pexels-photo-8549835.jpeg?auto=compress&cs=tinysrgb&dpr=2&h=650&w=940',
title: "Vase Image"
};
}
render() {
return (
<div>
<img src={this.state.url} alt={`${this.state.title}'s picture`} className="img-responsive" />
<span>Hello {this.state.name}</span>
</div>
);
}
}
render(<App />, document.getElementById('root'));
Let’s add some CSS code to resize our image.
# react
.img-responsive{
width: 90vw;
}
Output:
We can now see that we have easily added images in React.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Rana Hasnain Khan
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn