The hr Element in React
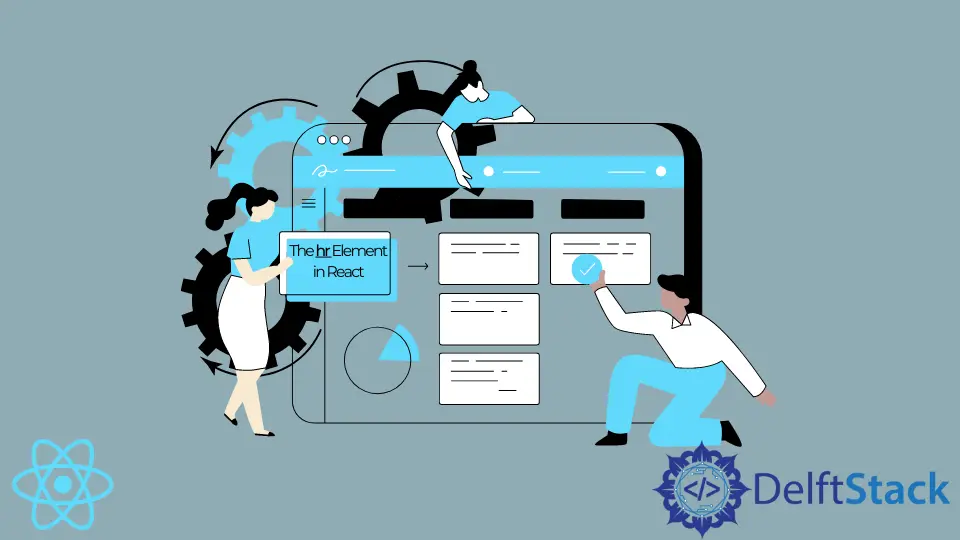
React is a component-based library that allows developers to build beautiful user interfaces in JavaScript. One of the biggest advantages of React is that you can re-use UI components, so you only have to write a minimal amount of code.
React also allows you to customize the styles and classes of every component and even to customize the fonts and colors of every instance of a component by passing dynamic values through props
.
Use the <hr>
Element for Section Dividers in React
Some web developers don’t know that in HTML, there is a separate <hr>
element for section dividers. It’s better to use separate HTML elements rather than relying on styles to feature a divider in your UI.
The <hr>
element does not look good without additional styles. If you want to feature this HTML element in your web application, you can use inline styles or define a separate class in your CSS file.
Let’s look at an example.
export default function App() {
return (
<div className="App">
Lorem ipsum
<Divider
primaryColor="red"
secondaryColor="grey"
heightValue={20}
></Divider>
Lorem Ipsum
</div>
);
}
function Divider(props) {
const { primaryColor, secondaryColor, heightValue } = props;
console.log(heightValue);
return (
<hr
style={{
color: primaryColor,
backgroundColor: secondaryColor,
height: heightValue
}}
/>
);
}
In this example, we have a custom Divider
component, which accepts three props: primaryColor
, secondaryColor
, and heightValue
. We use the style
attribute within the child component to write inline styles in React.
To set inline styles, we use curly braces to interpret the code inside them as valid JavaScript. Within the first pair of curly braces, we place an object, so ultimately we end up with two opening and closing curly braces.
This way, we can access the values from the prop
object and use them as values to style our element. Note that the property names like backgroundColor
are not the same as CSS properties like background-color
.
The words are combined and camel-cased.
This way, you can have multiple <hr>
dividers in your application. You can customize the element’s color, size, and other visual properties through passing props.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn