How to Access a DOM Element in React
- Understanding the Role of the DOM in React
- Using Refs to Access DOM Elements
- Accessing DOM Elements with the useEffect Hook
- Managing Focus in Class Components
- Conclusion
- FAQ
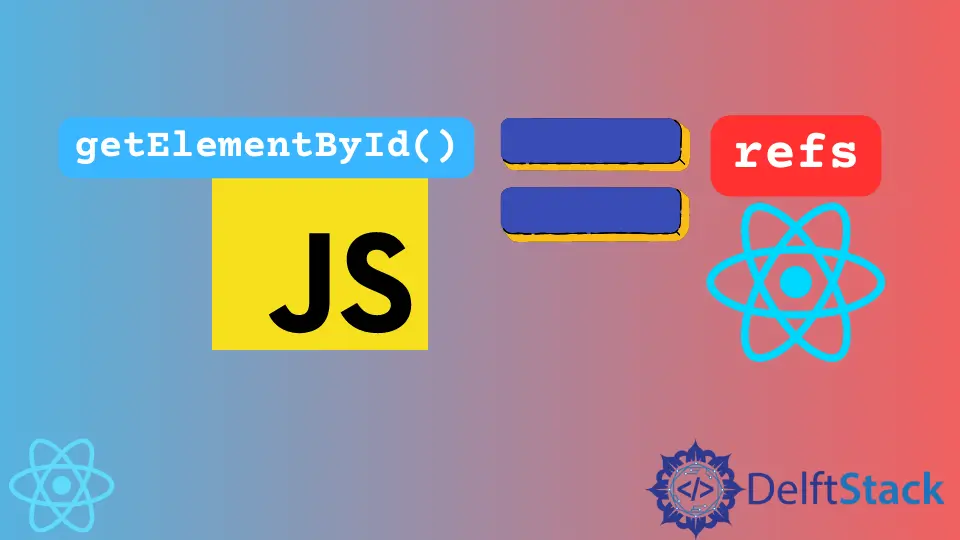
Accessing a DOM element in React can seem daunting, especially if you’re used to traditional JavaScript methods. However, React provides a modern approach that simplifies DOM manipulation while maintaining the integrity of its virtual DOM.
In this article, we will explore various methods to access DOM nodes in React, including the use of refs, the useEffect
hook, and more. By the end of this guide, you’ll have a solid understanding of how to effectively interact with DOM elements in your React applications, making your web development experience smoother and more efficient.
Understanding the Role of the DOM in React
The Document Object Model (DOM) represents the structure of a webpage. In traditional web development, accessing and manipulating the DOM directly was common practice. However, React’s virtual DOM changes the game by creating a lightweight copy of the actual DOM. This allows React to optimize updates and rendering processes. While React encourages developers to avoid direct DOM manipulation, there are scenarios where you might need to access a DOM element directly, such as focusing an input field or integrating with third-party libraries.
Using Refs to Access DOM Elements
One of the most straightforward ways to access a DOM element in React is by using refs. Refs provide a way to reference DOM nodes directly. This is particularly useful for cases where you need to interact with a DOM element, such as focusing an input field or measuring its size.
Here’s a simple example of how to use refs in a functional component:
import React, { useRef } from 'react';
const FocusInput = () => {
const inputRef = useRef(null);
const handleFocus = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" placeholder="Click the button to focus" />
<button onClick={handleFocus}>Focus Input</button>
</div>
);
};
export default FocusInput;
Output:
The input field is focused when the button is clicked.
In this example, we create a functional component called FocusInput
. We use the useRef
hook to create a reference to the input element. When the button is clicked, the handleFocus
function is invoked, which calls focus()
on the input element referenced by inputRef
. This demonstrates how refs allow us to directly interact with DOM elements in a React application.
Accessing DOM Elements with the useEffect Hook
Another effective way to access DOM elements in React is by utilizing the useEffect
hook. This hook allows you to perform side effects in your components, such as interacting with the DOM after the component mounts. It’s particularly useful for scenarios where you need to access a DOM element once the component is rendered.
Consider the following example, where we change the background color of a div after the component mounts:
import React, { useEffect, useRef } from 'react';
const ColorChangeDiv = () => {
const divRef = useRef(null);
useEffect(() => {
divRef.current.style.backgroundColor = 'lightblue';
}, []);
return (
<div ref={divRef} style={{ height: '100px', width: '100px' }}>
Color Change Div
</div>
);
};
export default ColorChangeDiv;
Output:
The div's background color changes to light blue after the component mounts.
In this example, the ColorChangeDiv
component uses useRef
to reference a div element. The useEffect
hook runs after the component mounts, changing the div’s background color to light blue. This showcases how the useEffect
hook can be leveraged to access and manipulate DOM elements effectively.
Managing Focus in Class Components
While functional components are increasingly popular, many applications still use class components. Accessing DOM elements in class components is also straightforward using refs.
Here’s an example of how to manage focus in a class component:
import React, { Component } from 'react';
class FocusInputClass extends Component {
constructor(props) {
super(props);
this.inputRef = React.createRef();
}
handleFocus = () => {
this.inputRef.current.focus();
};
render() {
return (
<div>
<input ref={this.inputRef} type="text" placeholder="Click the button to focus" />
<button onClick={this.handleFocus}>Focus Input</button>
</div>
);
}
}
export default FocusInputClass;
Output:
The input field is focused when the button is clicked.
In this class component, we create a ref using React.createRef()
in the constructor. The handleFocus
method is called when the button is clicked, focusing the input field. This example illustrates that accessing DOM elements in class components is just as easy as in functional components.
Conclusion
In this article, we explored various methods to access DOM elements in React, including using refs and the useEffect
hook. While React promotes a declarative approach to UI development, there are instances where direct DOM manipulation is necessary. By understanding how to use refs and lifecycle methods effectively, you can enhance user interactions in your React applications. Remember, while accessing the DOM directly can be useful, it’s essential to leverage React’s strengths to maintain performance and readability.
FAQ
-
What is a ref in React?
A ref is a way to directly reference a DOM element in React, allowing you to interact with it programmatically. -
When should I use refs?
Use refs when you need to manage focus, trigger animations, or integrate with third-party libraries that require direct DOM access. -
Can I use refs with functional components?
Yes, you can use refs with functional components by utilizing theuseRef
hook. -
How does the useEffect hook work?
TheuseEffect
hook allows you to perform side effects in functional components, such as accessing or manipulating the DOM after the component has rendered. -
Is it necessary to manipulate the DOM directly in React?
No, it’s generally best to avoid direct DOM manipulation in React. However, there are specific scenarios where it may be required.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn