How to Create a Sticky Footer in React
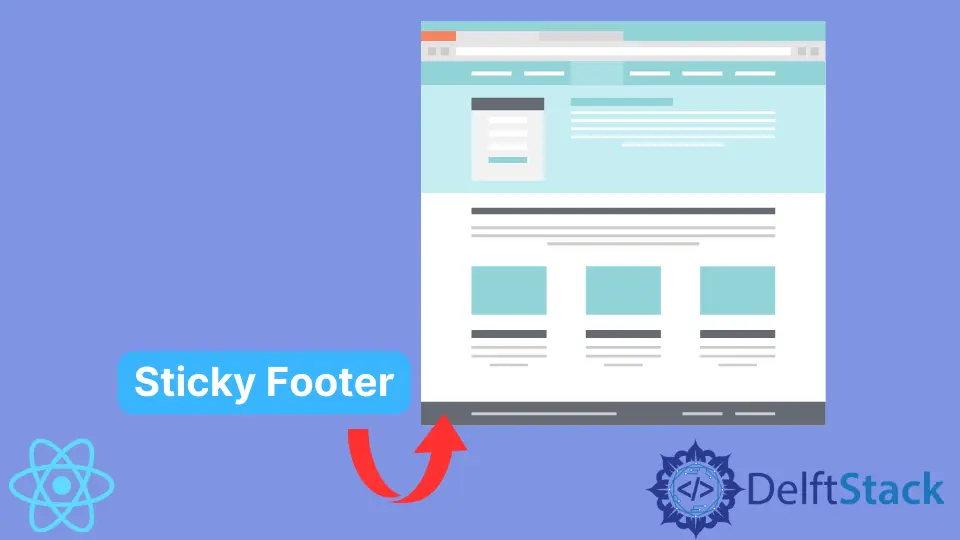
We often need to declare or divert users’ attention to something important when developing modern applications.
It can be achieved by creating a sticky footer. As the name suggests, sticky footers stick to the bottom of the users’ view and stay there even if the users scroll.
Sticky footers are particularly useful if you have a long scrollable page but want to display a UI before users reach the bottom of the page.
With a bit of CSS magic and basic HTML knowledge, you can create a sticky footer in React as well.
Using Regular CSS and React to Create a Sticky Footer in React
Creating a sticky footer in React is more complex than simply creating a div
and fixing its position to the bottom; setting a position:fixed
property on a div
is a good start, but not enough.
The problem with the fixed
value is that it removes the element from the normal document flow. Therefore, if there’s any UI element or text at the bottom of your page, it will overlap with the sticky div
.
Despite this shortcoming, position:fixed
is still necessary to create a sticky footer.
The solution is to create a wrapper div
(a div
element that takes up space in the document flow). Creating such a div
allows you to reserve space for the sticky footer to not overlap with any regular HTML elements.
Let’s look at the code:
function StickyFooter({ children }) {
let regularDiv = {
backgroundColor: "black",
borderTop: "2px solid red",
textAlign: "center",
padding: "40px",
position: "fixed",
left: "0",
bottom: "0",
height: "80px",
width: "100%",
}
let wrapperDiv = {
display: 'block',
padding: '40px',
height: '80px',
width: '100%',
}
return (
<div style={{height: "200vh"}}>
<div style={wrapperDiv} />
<div style={regularDiv}>
{ children }
</div>
</div>
)
}
Here, we define a sticky div
and a wrapper div
to reserve space for it. This way, the sticky div
won’t cover any part of your page.
You can also destructure the props, like in the example. Any elements you place between the opening and closing tags of the StickyFooter
component will go inside the sticky footer.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn