How to Export Function in React
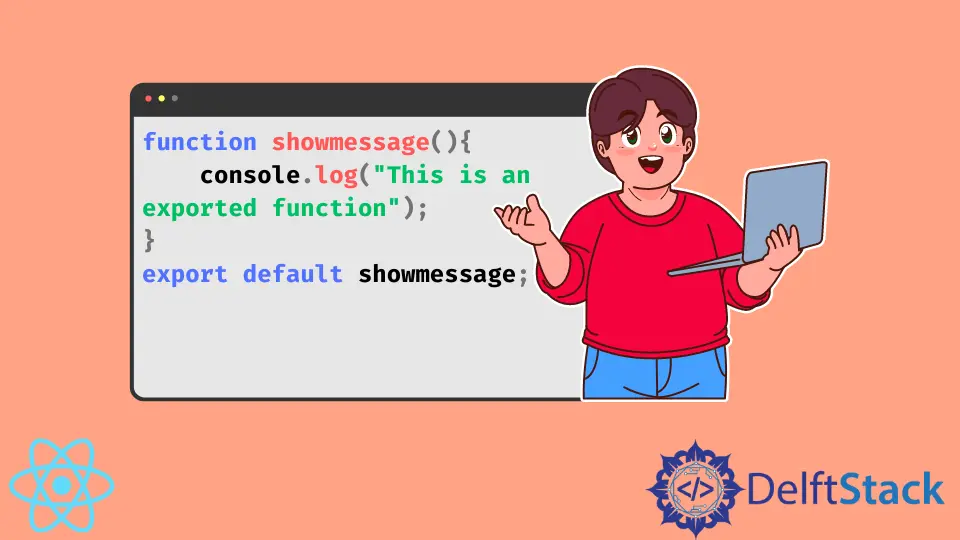
Sometimes we need to create a library that contains necessary variables and functions. The question is how we can get the variables and functions inside a library and use them on another script.
The answer is the use of the export
keyword. Now the export
keyword here will specify the sharable elements of a library.
This brief article will show how we can export functions in React JS. Also, we will see necessary examples and explanations to make the topic easier.
Use the export
Function in React
We created a separate JS file in the below example, and inside the file, there is a function named showmessage()
. We declared the showmessage()
function as a default export
.
Code - Export.js
:
function showmessage() {
console.log('This is an exported function !!!');
}
export default showmessage; // Exporting the function
Take a look at our main JS file, where we exported the function showmessage()
for our external JS file. This code for our main JS file will look like the one below.
Code - App.js
:
import './App.css';
import showmessage from './Export';
function App() {
const handleClick = (e) => {
e.preventDefault();
showmessage();
}
return (
<a href='#' onClick={handleClick}>
Click me
</a>
);
}
export default App;
In the above code, we first imported necessary files, and inside the main function, we created a handleClick
. Inside the handleClick
, we just passed the function showmessage()
, which we imported from our external JS file.
When you run the code above, you’ll get the below output in your web console.
This is an exported function !!!
The example codes shared in this article are written in React JS project. Your system must contain the latest Node JS version to run a React project.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn