How to Display JSON in React
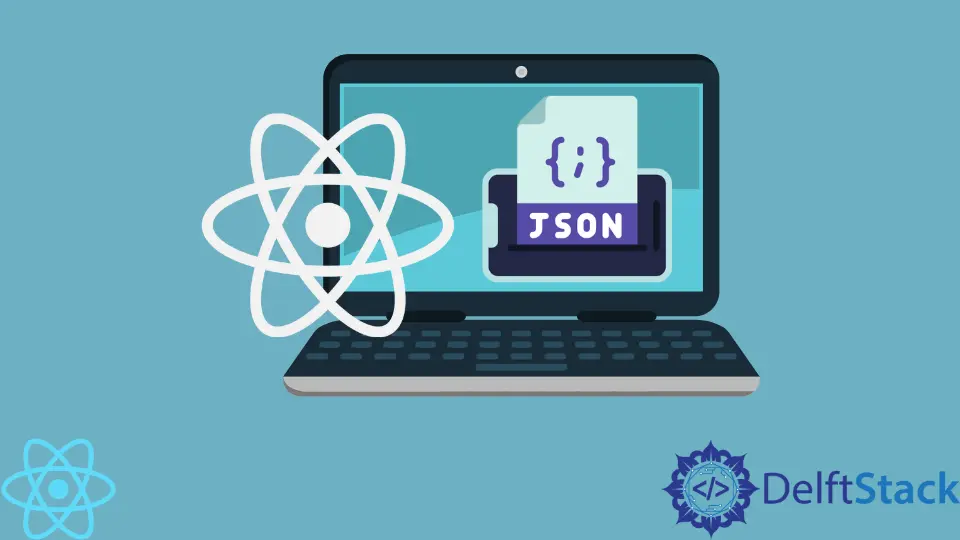
When building web applications in React, it is a common necessity to receive external data and display it. JSON (JavaScript Object Notation) is a format commonly used to share data stored as objects.
The focus of this article will be to show how to display JSON-formatted data in React.
If you need to print neatly formatted JSON data, you must understand two concepts: the JSON.stringify()
method and the <pre>
element in HTML.
the JSON.stringify()
Method
This method takes valid JavaScript objects and converts them into JSON formatted strings. It can take up to three arguments.
However, for this article, we only need to understand the first argument, which takes valid JavaScript objects and converts them to JSON strings.
JSON.stringify(value)
The method returns the value
argument (JavaScript object) formatted as a string. The returned value is formatted to be a readable JSON string; however, displaying it to retain readability can be challenging.
the <pre>
Tag in HTML
Front-end developers use the <pre>
tag to retain the formatting of the text. Letters in the text have equal widths.
It also retains spaces and line breaks in the original text wrapped with this tag.
If you use JSON.stringify()
to convert data into a JSON string, you need to wrap the returned value with <pre>
tags.
Display JSON in React
Here is how to take a JavaScript object and convert it into a JSON string, and retain neat formatting:
export default function App() {
const data = { name: "Irakli", age: 24, height: 185 };
return (
<div className="App">
<p>{JSON.stringify(data)}</p>
<pre>{JSON.stringify(data)}</pre>
</div>
);
}
Open the CodeSandbox live demonstration to see code in effect.
The live demo contains the JSON string with and without the <pre>
tag. It can help you visually understand the difference between formatted and not formatted text.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn