How to Allow CORS in React
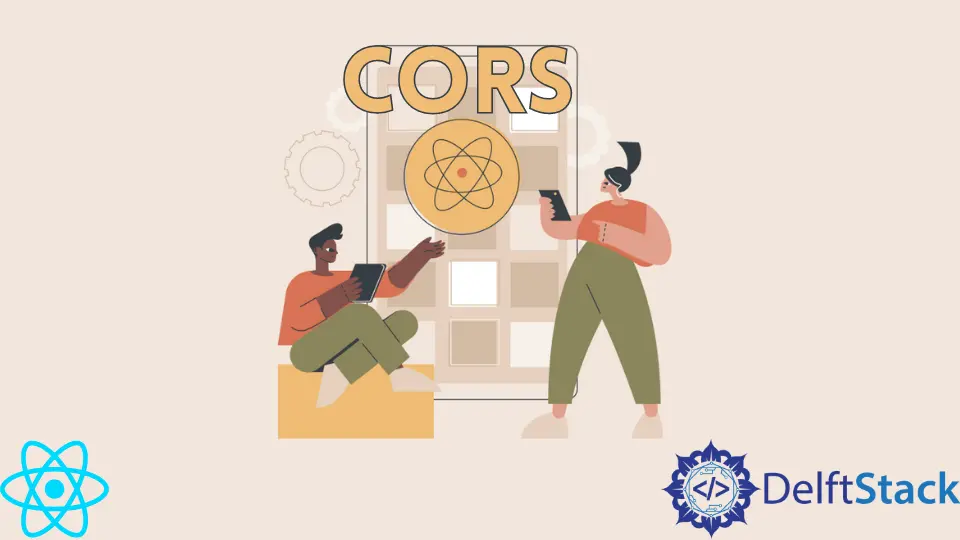
We will introduce CORS and how to allow it in React.
CORS in React
Developers have struggled with CORS because it’s a tricky concept, like for new developers who recently started working with third-party API from single-page applications on React.
CORS stands for cross-origin resource sharing; it’s a protocol like HTTPS that defines rules of sharing resources from a different origin. It enables us to access a resource from a different origin and overrides our browser’s default behavior due to SOP.
When a client requests a resource, the response contains a stamp that tells our browser to allow resource sharing across different origins.
Once our browser identifies this stamp, the responses for requests that originate from different origins are allowed to pass through.
Let’s have an example using one API named https://jsonplaceholder.typicode.com
.
We can enable CORS by adding an API proxy in package.json
. We will go to package.json
and add "proxy": "https://jsonplaceholder.typicode.com"
.
The package.json
will look like this.
# react
{
"name": "react",
"version": "1.0.0",
"description": "React example starter project",
"keywords": ["react", "starter"],
"proxy": "https://jsonplaceholder.typicode.com",
"main": "src/index.js",
"dependencies": {
"react": "17.0.2",
"react-dom": "17.0.2",
"react-scripts": "4.0.0"
},
"devDependencies": {
"@babel/runtime": "7.13.8",
"typescript": "4.1.3"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test --env=jsdom",
"eject": "react-scripts eject"
},
"browserslist": [">0.2%", "not dead", "not ie <= 11", "not op_mini all"]
}
React CORS is enabled, and the API works fine without errors.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn