How to Generate a PDF From React Component
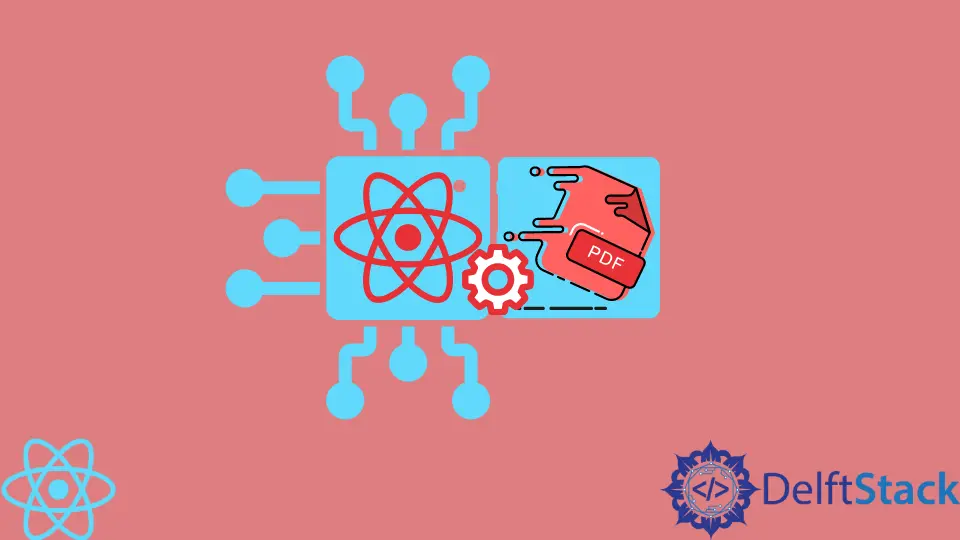
We occasionally need to produce a PDF file from React components. This tutorial will look at creating a PDF file from React components.
Generate a PDF From React Component
React is a widely used front-end development framework. We occasionally need to produce PDF documents while designing online apps, and it might be a whole web page or only a component.
We may use the jspdf
and react-dom/server
libraries to produce a PDF file using React components.
To begin, use the following command to install the jspdf
npm package.
npm install jspdf --save
By default, react-dom/server
includes projects generated by Create React App.
Following the installation, you must import that package into your appropriate React component.
import JsPDF from 'jspdf';
import ReactDOMServer from 'react-dom/server';
Now, we will develop a function to produce and export PDFs. Let’s see the full example:
import jsPDF from 'jspdf';
import React from 'react';
import ReactDOMServer from 'react-dom/server';
const document = new jsPDF();
const Shiv =
<b>You have Downlaoded a component from react<
/b>;
export default function App() {
const save = () => {
document.html(ReactDOMServer.renderToStaticMarkup(Shiv), {
callback: () => {
document.save("yourDoc.pdf");
},
});
};
return (
<div>
<button onClick={save}>React Component to pdf</button><
/div>
);
}
We created a new jsPDF
function object instance and assigned it to document
. Then, there’s the Shiv
JSX statement, which displays some bold text.
Following that, in App()
, we write the save
function, which calls the document.html
method using the HTML string generated by the renderToStaticMarkup
method.
renderToStaticMarkup
is useful when stripping out the extra characteristics and might save some bytes if you want to utilize React as a primary static page generator. Do not use this approach if you’re utilizing React on the client to make the markup interactive.
Instead, use ReactDOM and renderToString
on the server.
Output:
You can see a button that will help download a React component in pdf. When you click on it, it will download a file called yourDoc.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn