How to Use CLSX to Conditionally Apply Classes in React
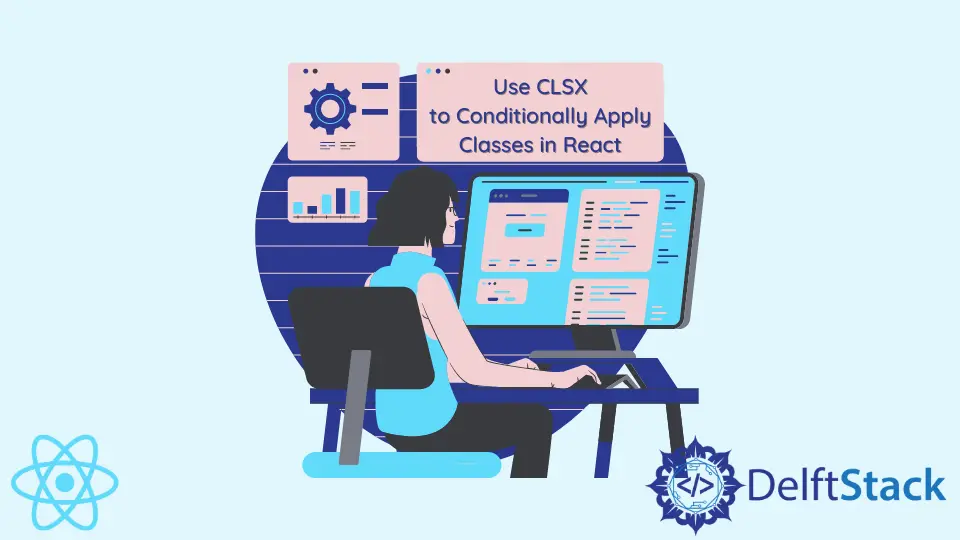
This article will explain the clsx()
function and apply classes in React conditionally.
the clsx()
Function in React
clsx()
function from the package of the same name is a JavaScript utility used to set up conditions for setting the value of the className
attribute. It accepts an unlimited number of arguments not limited to one specific type.
Ultimately, the clsx()
function returns a string
interpolation that checks the boolean
value of JavaScript variables and applies classes accordingly.
It’s certainly possible to write these strings yourself, but it’s much more time-consuming than simply using the clsx()
function.
Check out the official npm docs.
Use the clsx()
Function to Set Conditional Classes in React
React developers use the clsx()
function to apply classes.
We can set up a condition that needs to be satisfied to apply a specific class, but it’s not a requirement.
Example:
import "./styles.css";
import clsx from "clsx";
const classNameOne = "redButton";
const classNameTwo = "blueBorder";
export default function App() {
const number = 3;
return (
<div className="App">
<button className={clsx(classNameOne, { [classNameTwo]: number > 5 })}>
A sample button
</button>
</div>
);
}
In the example above, we have two variables with string type values. We also have one variable, called number
, set to an arbitrary number to demonstrate how the clsx()
function conditionally applies (or doesn’t apply) to the specified class.
The first argument to the function classNameOne
doesn’t have any associated conditions, so it is often applied no matter what. A second argument is an object with key-value
pair of the classNameTwo
property and the condition as its value.
In this case, the number
variable has a value of 3, which isn’t more than 5, so the classNameTwo
doesn’t apply. Try changing the value on CodeSandbox and see what happens for yourself.
This is an example of basic conditional styling. At first glance, it’s not apparent why you need the clsx()
function, but once your conditions for styling become more complex, clsx()
allows you to set these conditions without difficulty.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn