The cloneElement Method in React
-
What Is
cloneElement()
in React -
props.children
vsReact.cloneElement()
in React -
React.cloneElement()
Syntax in React -
When to Use
children
Prop in React
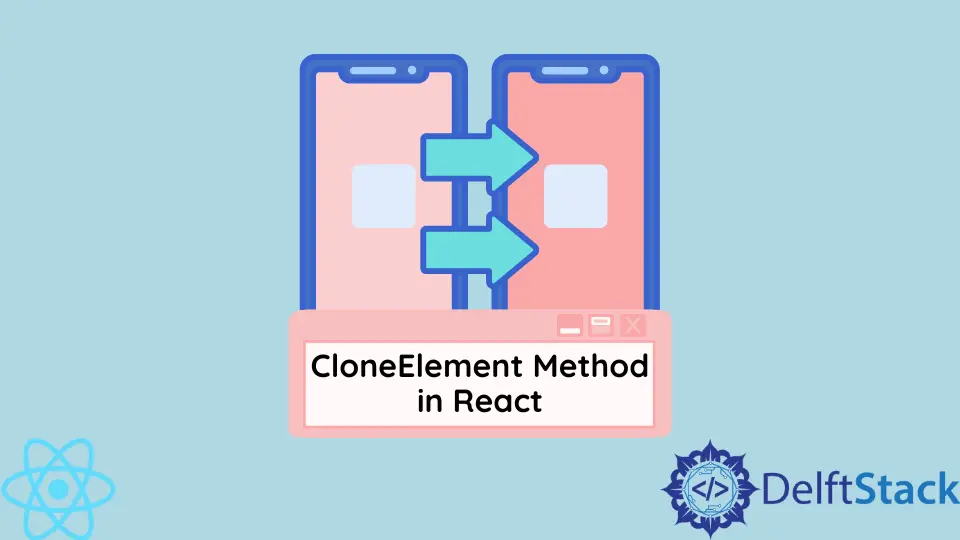
This article will explore how props.children
is associated with React.cloneElement()
and explain their similarities and differences.
What Is cloneElement()
in React
In React, we use props
to manually pass down the data from parent components to the children.
At first glance, it may seem that props
are straightforward, but if you take a look at the props
object, you’ll notice that it’s more complex than it looks.
For instance, you’ll notice the children
property, even though you might not have explicitly set a children
attribute.
The cloneElement()
is one method that makes up the core React
library. As the name suggests, React.cloneElement()
is used to clone a React element and typically build on top of the original component.
For instance, it allows you to add props
and modify props.children
value of a component.
props.children
vs React.cloneElement()
in React
Many React developers use the two interchangeably, but in reality, props.children
and React.cloneElement()
are useful for different purposes.
The props.children
is used to access all of the content passed between opening and closing tags of the child component when it is invoked. The only drawback is that you can’t modify props.children
in any way.
In React, props are immutable, and that rule also includes the children
property.
That’s when React.cloneElement()
comes into play. You can use it within the parent component to add certain props
, modify the children, or extend the functionality of children components in any other way, such as adding an event handler or a ref
attribute to facilitate the manipulation of DOM.
React.cloneElement()
Syntax in React
Before using React.cloneElement()
in your projects, you should understand its syntax: what kind of arguments it takes, their structure, and what they represent.
The first argument to the React.cloneElement()
method is the element or component you want to clone (or build on top of).
The method automatically preserves all original props
of the component, but you can add more props
as a second argument to the method, which is supposed to be an array.
The third argument to the method is used to specify the children
property of the cloned object. Unlike regular props
, children
of the original component is not preserved in the copy.
With that being said, let’s look at the actual example.
export default function App() {
return (
<div className="App">
<Child text={"Hello, World"}>Goodbye</Child>
{React.cloneElement(<Child></Child>, { text: "Hello, Universe" }, "Ciao")}
</div>
);
}
function Child(props) {
return (
<div>
<h1>{props.text}</h1>
<h1>{props.children}</h1>
</div>
);
}
In this example, we have a simple demonstration of the utility of the React.cloneElement()
method.
If you look in the live CodeSandbox demo, you’ll see that we have two <Child>
components in the parent App
component, one original and one copy.
According to the syntax we described before, the first argument is the component we are trying to copy, <Child>
. A second argument is an object with a key-value pair representing a prop
attribute and its value.
In this case, the props of the original component are preserved, but we are overwriting the value of the text
. The last argument is a string, which will place props.children
value in the child component.
When to Use children
Prop in React
You can often get away with using props.children
to access the values passed between the opening and closing tags of the child component in JSX.
The children
prop is particularly useful if the content of your child component is dynamically generated, for instance, a message box.
However, one important detail to remember is the props.children
value is only a descriptor of the values. You are not accessing the values, only their representation when you access it.
For this reason, you can’t change props.children
or any other props; you can only read their value.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn