Button onClick Event in React
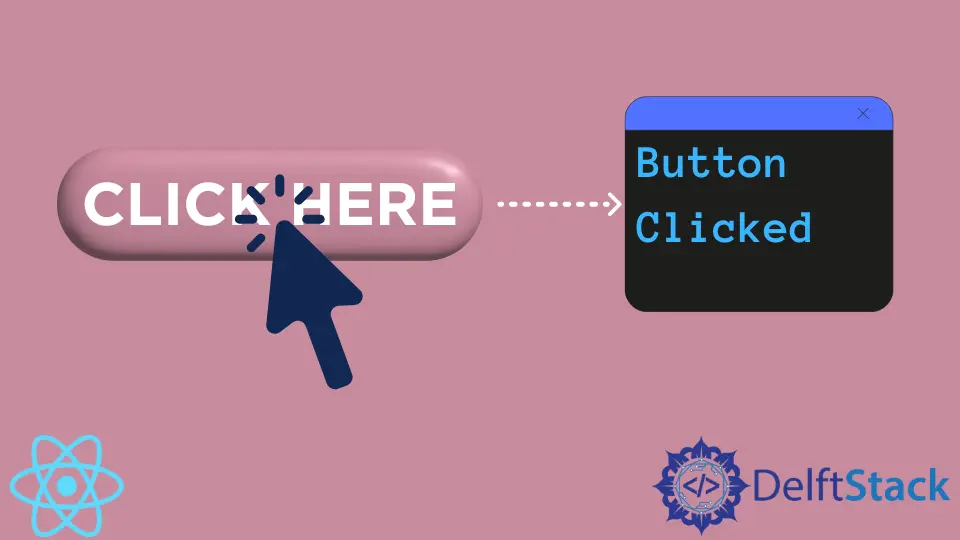
We will introduce using the button onClick event
in React.
Use onClick
Event in a Button in React
When developing a web application or commercial software, we need to use onClick
events to perform many functions.
This tutorial will go through an example and create a new React application with a button with an onClick
event.
So let’s create a new React application by using the following command.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Let’s run our app to check if all dependencies are installed correctly.
# react
npm start
Now, we will return a button in App.js
with a onClick
event of function h()
.
# react
<button onClick={() => h()}>Click This Button </button>;
Let’s create the function h()
that will console.log
a value.
# react
function h() {
console.log("Button Clicked");
}
Let’s add some styles to our buttons using CSS.
# react
button {
background: black;
color: white;
border: none;
padding: 15px 15px;
}
button:hover {
background: white;
color: black;
border: 1px solid black;
}
Output:
We can easily create any onClick
events on buttons and pass any functions using these simple steps. Check the code here.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn