How to Build a Date Picker Using the React-Bootstrap Library
- Setting Up Your React Environment
- Creating the Date Picker Component
- Integrating the Date Picker in Your App
- Customizing the Date Picker
- Conclusion
- FAQ
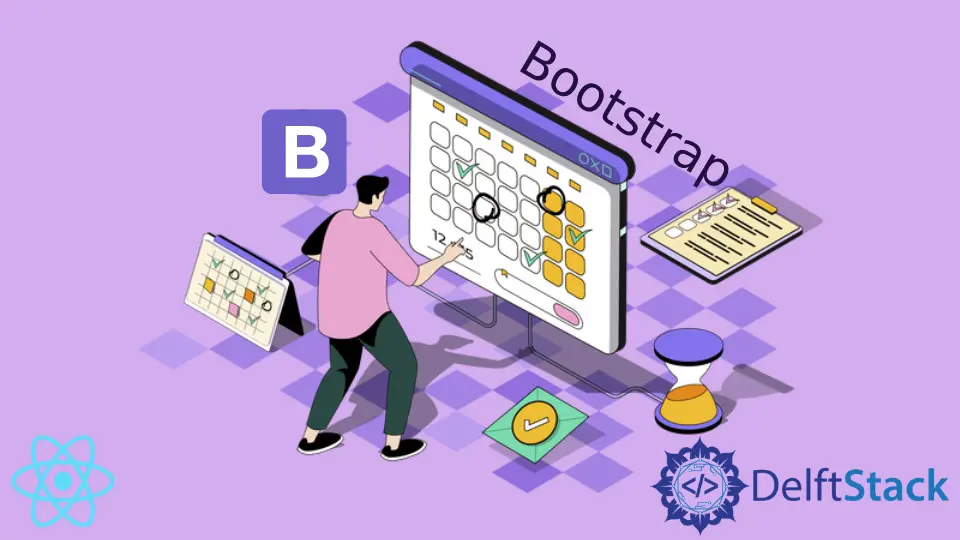
Creating a user-friendly date picker is essential for many web applications. If you’re using React and want to enhance your forms with a stylish and functional date picker, the React-Bootstrap library is a fantastic choice.
This article will guide you through the process of building a date picker using the Form component from the React-Bootstrap library. We’ll explore how to set up your environment, implement the date picker, and customize it to meet your needs. Whether you are a beginner or an experienced developer, this guide will provide valuable insights and practical examples to help you create a seamless date selection experience for your users.
Setting Up Your React Environment
Before we dive into building the date picker, it’s important to ensure that you have the right environment set up. You’ll need Node.js and npm installed on your machine. If you haven’t done this yet, head to the official Node.js website to download and install the latest version.
Once you have Node.js and npm ready, you can create a new React application using Create React App. Open your terminal and run the following command:
npx create-react-app my-date-picker
Next, navigate into your project directory:
cd my-date-picker
Now, let’s install the React-Bootstrap library along with Bootstrap itself. Run the following command:
npm install react-bootstrap bootstrap
After installing these packages, you need to include Bootstrap CSS in your project. Open the src/index.js
file and add the following import statement at the top:
import 'bootstrap/dist/css/bootstrap.min.css';
This setup will allow you to use React-Bootstrap components seamlessly in your application. With everything in place, you’re ready to start building your date picker.
Creating the Date Picker Component
Now that your environment is set up, let’s create the date picker component. In your src
folder, create a new file named DatePickerComponent.js
. This file will contain the logic and UI for your date picker.
Here’s a simple implementation using the Form component from React-Bootstrap:
import React, { useState } from 'react';
import { Form } from 'react-bootstrap';
const DatePickerComponent = () => {
const [date, setDate] = useState('');
const handleDateChange = (event) => {
setDate(event.target.value);
};
return (
<Form>
<Form.Group controlId="datePicker">
<Form.Label>Select a Date</Form.Label>
<Form.Control
type="date"
value={date}
onChange={handleDateChange}
/>
</Form.Group>
</Form>
);
};
export default DatePickerComponent;
In this code, we first import React and the necessary components from React-Bootstrap. We use the useState
hook to manage the selected date’s state. The handleDateChange
function updates the state whenever the user selects a new date.
The Form.Control
component is set to type “date,” which gives us a native date picker in modern browsers. This is a simple and effective way to create a date picker without the need for additional libraries.
This component is straightforward and leverages the built-in capabilities of HTML5 input types. However, if you want more customization or features, you can explore additional libraries like react-datepicker
or react-datetime
.
Integrating the Date Picker in Your App
Now that we have our DatePickerComponent
, it’s time to integrate it into our main application. Open the src/App.js
file and update it to include the date picker.
Here’s how you can do it:
import React from 'react';
import DatePickerComponent from './DatePickerComponent';
const App = () => {
return (
<div className="container mt-5">
<h1>Date Picker Example</h1>
<DatePickerComponent />
</div>
);
};
export default App;
In this code, we import our DatePickerComponent
and include it in the main App
component. The container mt-5
classes from Bootstrap add some margin to the top, giving it a nicer layout.
With this integration, you now have a fully functional date picker within your application. Users can select a date, and the state will update accordingly. This is a solid foundation, and you can expand upon it by adding validations, formatting, or even integrating it with other form components.
Customizing the Date Picker
While the basic date picker is functional, you might want to customize it further to fit your application’s needs. React-Bootstrap allows for several enhancements, such as adding validation, custom styling, and more.
To add validation, you can modify the handleDateChange
function to include checks, ensuring that the selected date is within a specific range or meets certain criteria. For instance, you could prevent users from selecting past dates:
const handleDateChange = (event) => {
const selectedDate = event.target.value;
const today = new Date().toISOString().split('T')[0];
if (selectedDate < today) {
alert("Please select a future date.");
} else {
setDate(selectedDate);
}
};
This code snippet checks if the selected date is less than today’s date and alerts the user if it is. This simple validation enhances user experience by guiding them to make valid selections.
Output:
Users will be alerted if they select a past date.
Furthermore, you can style your date picker by applying custom CSS classes or using Bootstrap’s utility classes. This allows you to create a date picker that not only functions well but also looks great in your application.
Conclusion
Building a date picker using the React-Bootstrap library is a straightforward process that can significantly enhance your forms. With just a few lines of code, you can create a functional and stylish date picker that improves user experience. By following the steps outlined in this article, you can easily integrate a date picker into your React application. Remember, customization is key, so feel free to explore additional features and styles to make the date picker truly yours.
FAQ
-
What is React-Bootstrap?
React-Bootstrap is a popular library that provides Bootstrap components as React components, allowing developers to use Bootstrap styles and functionality seamlessly in React applications. -
Can I customize the date picker?
Yes, you can customize the date picker by adding validation, custom styles, and additional features to meet your application’s requirements. -
Do I need to install any additional libraries for a date picker?
The basic date picker can be created using HTML5 input types, but for more advanced features, you might consider using libraries like react-datepicker. -
How do I handle date validation?
You can handle date validation by checking the selected date against certain criteria, such as ensuring it is not a past date or falls within a specific range. -
Is the date picker accessible?
Yes, using the Form component from React-Bootstrap ensures that the date picker is accessible, but you should always test for accessibility compliance in your application.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn