How to Add Multiple classNames to React Component
-
Use the Template Literals Method to Add Multiple
classNames
to React Component -
Use the
classnames
Package to Add MultipleclassNames
to React Component -
Use the
classnames
Package and the.map
Method to Add MultipleclassNames
to React Component - Conclusion
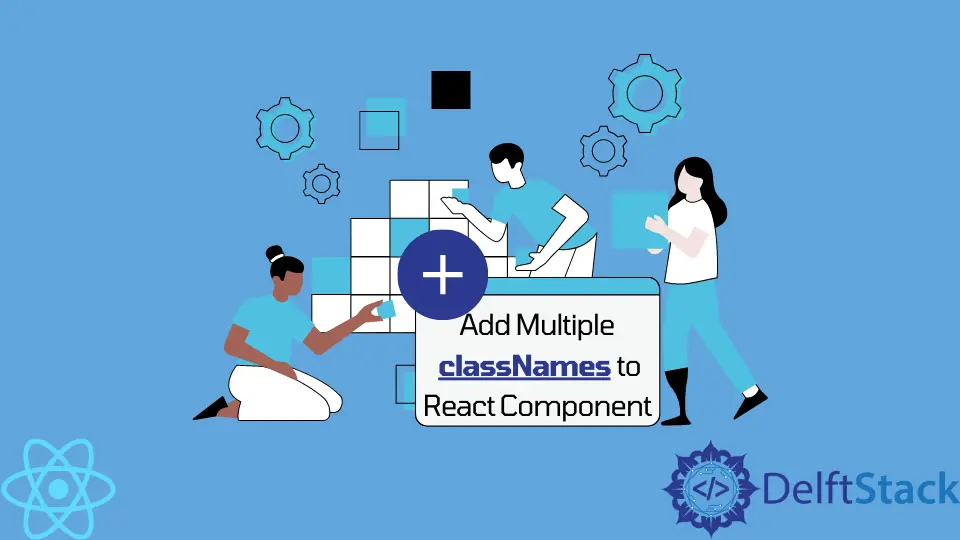
classNames
in React perform the same function as classes in JavaScript. They are used instead of classes because class
is a reserved word in React.
Adding multiple classNames
to a component gives us room to set some conditions for that component. One className
is used to set the styling; the other is used to set the conditions.
This is ideal when we are creating web pages that will have buttons.
Additionally, we might want to add extra styling to a component to overwrite the original styling in certain situations. Adding an extra className
allows us to do this smoothly.
Besides, using multiple classNames
to set styling makes CSS files easier to read, track, and adjust since a single className
could become overloaded with too many stylings. Also, in situations where multiple CSS classes have similar attributes, we should create another class for similar attributes for ease of corrections and add-ons.
Let us look at different ways to add multiple classNames
to a component.
Use the Template Literals Method to Add Multiple classNames
to React Component
The template literals, otherwise called the template strings, allow users to create and manipulate multiple expressions wrapped in backticks, followed by the $
sign and curly braces.
We will create a button webpage example that shows the effect of multiple classNames
using CSS stylings alongside it. Navigate to the App.js
file of our project folder and write these codes:
Code Snippet- App.js
:
import React from "react";
import "./App.css";
export default function App() {
const [classNames, setClassNames] = React.useState(``);
const [showRed, setShowRed] = React.useState(false);
const [showBlue, setShowBlue] = React.useState(false);
React.useEffect(() => {
setClassNames(`${showRed ? "red" : ""} ${showBlue ? "blue" : ""}`);
}, [showRed, showBlue]);
return (
<div>
<button onClick={() => setShowRed(showRed => !showRed)}>
Toggle Red
</button>
<button onClick={() => setShowBlue(showBlue => !showBlue)}>
Toggle Light Blue
</button>
<div className={classNames}>hit me!</div>
</div>
);
}
The template literals are handy for accommodating classNames
when we call the setClassNames
functions. This is where we get to set our classes red
and blue
, respectively.
Then we will do a bit of coding inside the App.css
file:
Code Snippet- App.css
:
.red {
color: red;
}.blue {
background-color: lightblue;
width: 50px;
}
Output:
We used the button onClick
event listener to tell React what to do when either button is hit. As we hit one of the buttons, we can see one className
became active, and when we hit the other button, the other className
is invoked, and the colors change.
Use the classnames
Package to Add Multiple classNames
to React Component
One of the reasons why React is widely accepted is because it has unlimited room for potential developments and tweaking, thanks to its dependency packages.
The classnames
package is another method we can apply to add multiple classNames
to a component. It helps us write less code than the template literals method and is better applied when dealing with more than two classNames
.
Once we have created our project folder, we’ll navigate to the project folder still inside the internal and install the classnames
package with:
npm install classnames
Then, we start to code, starting with the App.js
file.
We are using the same styling as the first example. We won’t repeat the CSS snippet here.
Code Snippet- App.js
:
import React from "react";
import "./App.css";
const classNames = require("classnames");
export default function App() {
const [showRed, setShowRed] = React.useState(false);
const [showBlue, setShowBlue] = React.useState(false); return (
<div>
<button onClick={() => setShowRed(showRed => !showRed)}>
Toggle Red
</button>
<button onClick={() => setShowBlue(showBlue => !showBlue)}>
Toggle Light Blue
</button>
<div className={classNames({ red: showRed, blue: showBlue })}>
hello
</div>
</div>
);
}
Output:
The classnames
package is applied and wrapped in a div
, where we set the classNames
for each of our components, and then we use the onClick
event listener to change the states of each class.
We can see each className
gets toggled on/off when we click either of the buttons assigned to the classes.
Use the classnames
Package and the .map
Method to Add Multiple classNames
to React Component
If we want to apply the same styling to multiple classes simultaneously, we can use the classnames package alongside the .map
method. The .map
method is a JavaScript function that helps us carry a function across similar elements.
Once we have created our project folder, we’ll navigate to the project folder still inside the internal, and we will install the classnames
package with:
npm install classnames
Next, we proceed into coding; first, with the App.js
file:
Code Snippet- App.js
:
import React from "react";
import "./App.css";
const classNames = require("classnames");
export default function App() {
const [showRed, setShowRed] = React.useState(false);
return (
<div>
{["foo", "bar", "baz"].map(buttonType => (
<button
className={classNames({ [`btn-${buttonType}`]: showRed })}
onClick={() => setShowRed(showRed => !showRed)}
>
Button {buttonType}
</button>
))}
</div>
);
}
After declaring our classNames
, the .map
function maps all three classes to the buttonType
object. This will enable us to pass the same function across multiple classes.
Then, our App.css
will look like this:
.btn-foo,
.btn-bar,
.btn-baz {
color: red;
}
Output:
We assigned the same color to all the classes, and the onClick
event listener activated this CSS function when we toggled any buttons.
Conclusion
Working with multiple classes in React allows us to try different combinations, so we have different ways to work around an obstacle. And because it will enable us to carry out multiple stylings and conditions within a component, coding is much more flexible, and we can detect bugs and mistakes with pinpoint accuracy.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn