How to Get onKeyDown Event to Work With Divs in React
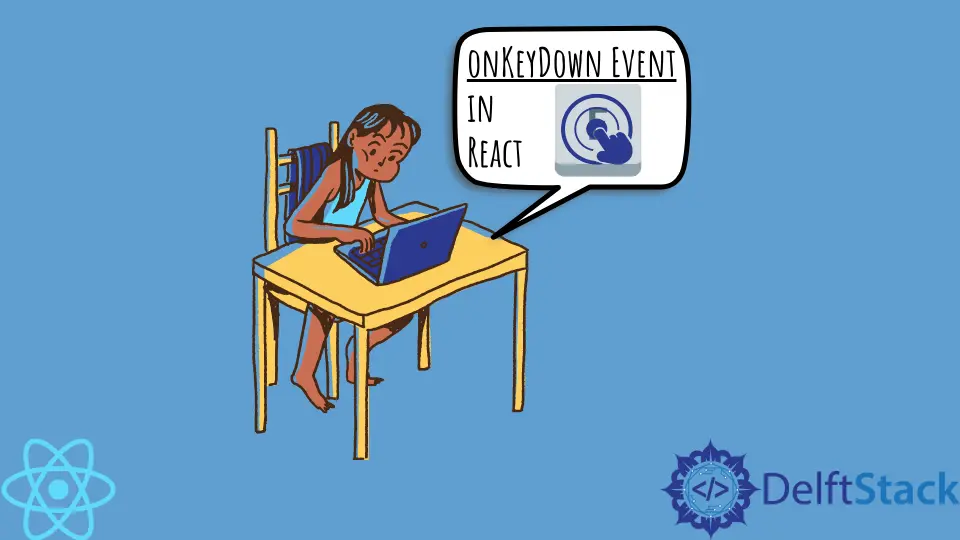
Handling events in React is the key to building modern web applications. One of the most useful events you can work with is the onKeyDown
event, which allows you to capture keyboard interactions within your components. While most developers are familiar with handling events in input fields or buttons, handling the onKeyDown
event on a div
can be incredibly powerful. This guide will walk you through the steps to effectively use the onKeyDown
event with div
elements in React, providing practical examples and explanations along the way. By the end, you will be equipped to enhance user interactions in your applications seamlessly.
Understanding the onKeyDown Event
The onKeyDown
event in React is triggered when a key is pressed down. Unlike onKeyPress
, which is deprecated, onKeyDown
captures all key presses, including special keys like Shift, Ctrl, and Escape. To utilize this event with a div
, you need to ensure that the div
is focusable. By default, div
elements are not focusable, so you will typically set the tabIndex
attribute to make them focusable.
Example 1: Basic onKeyDown with Div
Here’s a simple example of how to use the onKeyDown
event with a div
. In this example, we will create a div
that changes its background color when specific keys are pressed.
import React, { useState } from 'react';
const ColorChangingDiv = () => {
const [color, setColor] = useState('white');
const handleKeyDown = (event) => {
if (event.key === 'r') {
setColor('red');
} else if (event.key === 'g') {
setColor('green');
} else if (event.key === 'b') {
setColor('blue');
}
};
return (
<div
tabIndex="0"
onKeyDown={handleKeyDown}
style={{ width: '200px', height: '200px', backgroundColor: color }}
>
Press R, G, or B to change my color!
</div>
);
};
export default ColorChangingDiv;
Output:
Press R, G, or B to change my color!
In this example, we create a functional component called ColorChangingDiv
. The useState
hook initializes the background color to white. The handleKeyDown
function checks which key is pressed and updates the color accordingly. The tabIndex
attribute makes the div
focusable, allowing it to respond to keyboard events. The style
prop dynamically changes the background color based on the state.
Example 2: Handling Multiple Key Presses
In some scenarios, you might want to handle multiple key presses simultaneously. This can be achieved by modifying the handleKeyDown
function to accommodate combinations of keys. Here’s how you can do that.
import React, { useState } from 'react';
const MultiKeyDiv = () => {
const [message, setMessage] = useState('Press Ctrl + M');
const handleKeyDown = (event) => {
if (event.ctrlKey && event.key === 'm') {
setMessage('Ctrl + M pressed!');
}
};
return (
<div
tabIndex="0"
onKeyDown={handleKeyDown}
style={{ padding: '20px', border: '1px solid black' }}
>
{message}
</div>
);
};
export default MultiKeyDiv;
Output:
Press Ctrl + M
In this example, we create a MultiKeyDiv
component that listens for the Ctrl + M key combination. The handleKeyDown
function checks if the ctrlKey
property is true and if the key
pressed is ’m’. When both conditions are met, it updates the message displayed in the div
. This approach allows for more complex interactions and can enhance user experience significantly.
Conclusion
Using the onKeyDown
event with div
elements in React opens up a world of possibilities for creating interactive web applications. By understanding how to make div
elements focusable and capturing key presses, you can enhance user engagement and create dynamic interfaces. Whether you’re changing colors, displaying messages, or handling complex key combinations, the onKeyDown
event is a powerful tool in your React toolkit. Start experimenting with these examples in your projects and watch your applications come to life!
FAQ
-
How can I make a div focusable in React?
You can make a div focusable by adding thetabIndex
attribute with a value of “0”. -
Can I use the onKeyDown event with other HTML elements?
Yes, the onKeyDown event can be used with any focusable HTML element, including buttons, inputs, and custom components. -
What is the difference between onKeyDown and onKeyUp?
The onKeyDown event is triggered when a key is pressed down, while onKeyUp is triggered when the key is released. -
Is it possible to capture multiple key presses at once?
Yes, you can check the state of modifier keys (like Ctrl, Shift, or Alt) in combination with other keys in the onKeyDown event handler. -
Can I prevent default actions in the onKeyDown event?
Yes, you can callevent.preventDefault()
within the onKeyDown event handler to prevent the default action associated with the key press.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn