File Upload Component Styled With Material UI
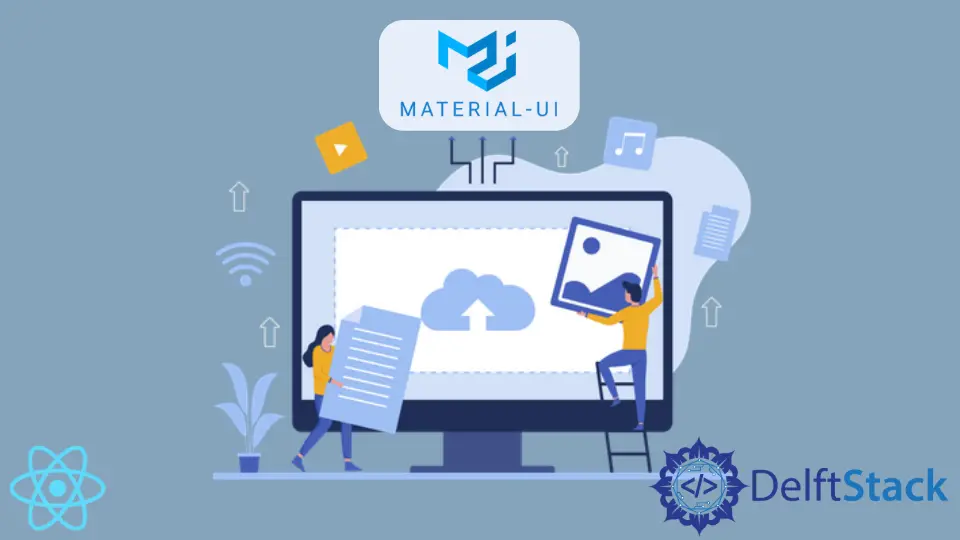
Many libraries allow you to build user interfaces for React applications. Material UI is one of the most popular and arguably the safest options.
The library provides custom components, such as buttons
, text fields
, and a robust API
that allows you to customize the look and behavior of your UI components.
There’s just one issue: Material UI doesn’t include any custom component for uploading files. This feature is often necessary, especially if you’re working on a React application that involves sharing photos and other media.
File Upload in Material UI in React
One way to create a glamorous file upload component with the styles of Material UI is to start with a regular HTML element
.
Let’s look at the actual code:
export default function App() {
return (
<div className="App">
<input type="file" />
</div>
);
}
As you can see on the live codesandbox demo, it doesn’t look glamorous.
That’s where Material UI components come in. The UI library includes existing styles, which you can use to improve the appearance of your <input>
element.
First, you must import a <Button>
custom component and wrap it around your unattractive file input element.
At this point, our code should look something like this:
export default function App() {
return (
<div className="App">
<Button variant="contained" component="label" color="primary">
{" "}
Upload a file
<input type="file" hidden />
</Button>
</div>
);
}
In this condition, our <Button>
component already looks much better. Using the variant
prop, we specified the type of the button.
The component
attribute in Material UI allows us to identify the HTML element used for the root node. In other words, by giving it a value of label
, we ensure that the Button
component will be compiled as a label
element in HTML.
Indeed, if you inspect the element to see the HTML structure, you’ll discover it is a <label>
element.
Finally, we give our button a default blue color.
To keep the functionality of the underlying <input>
HTML element but hide its unappealing visuals, we assign it the hidden
attribute. This way, when the user clicks on a button, the browser will prompt them to select a file.
It is a basic style of a custom Button
component in Material UI. Please look at its API documentation to learn more about customization possibilities.
For instance, you can use one of the icons in the Material UI library to make your button more attractive and intuitive.
export default function App() {
return (
<div className="App">
<Button variant="contained" component="label" color="primary">
{" "}
<AddIcon/> Upload a file
<input type="file" hidden />
</Button>
</div>
);
}
You can test it yourself on codesandbox.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn