How to Load Animated GIF in React
-
Use the
import
Statement to Add Loading Animated GIF in React -
Use the
require()
Function to Add Loading Animated GIF in React
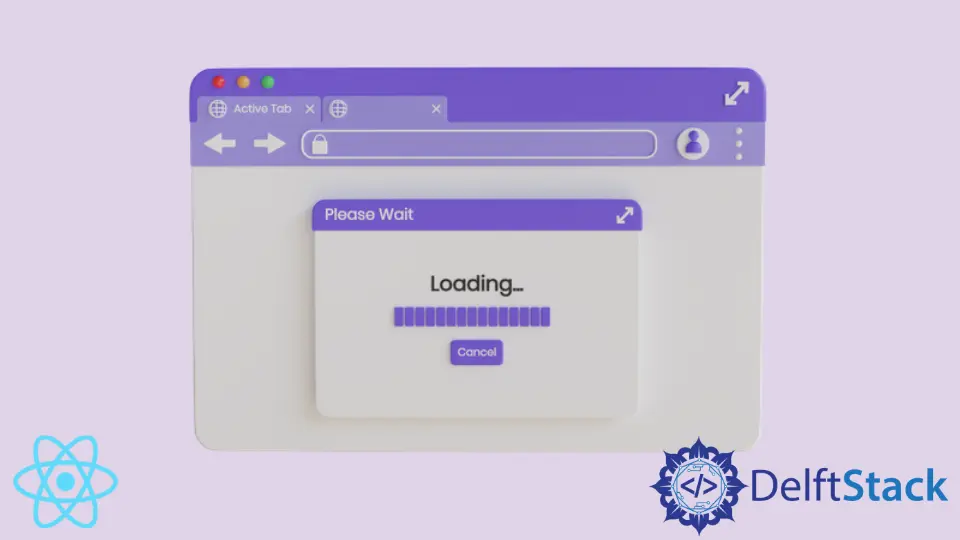
The saying that picture is worth a thousand words is true for web applications. In practice, you might need to use a live gif animation to emphasize points in your article, use gif elements in your logo, or other purposes.
Using visuals (such as symbols) to communicate the application’s state is widely accepted as a good UX practice. This article discusses using loading icon GIFs to better communicate that the data is loading and should wait.
Use the import
Statement to Add Loading Animated GIF in React
To feature a loading animated gif asset in your React application, first, you must turn it into a URI format. Uniform Resource Identifier is a data type used to represent visual assets as a string.
It enables React developers to use visuals like GIFs in web applications.
You must download the GIF file and store it in your project’s assets
or src
folder to use this method. If you’re using the create-react-app
package, you can use the import
statement to feature a visual resource at the specified path.
Let’s look at an example.
import loadingGif from "../assets/waiting.gif"
Tools in the create-react-app
environment automatically transform the .gif
file into URI. Then we can set the src
attribute equal to this value.
<img src={loadingGif} alt="wait until the page loads" />
Use the require()
Function to Add Loading Animated GIF in React
The import
statement was only introduced in ES6 and only worked in certain development environments.
If you’re limited to ES5 features, you can use the require()
function, which takes a path as an argument. The function transforms images into URI values.
Let’s look at the sample.
let loadingGif = require("../assets/waiting.gif")
<img src={loadingGif} alt="wait until the page loads" />
Ultimately, this expression will have the same effect as the import
statement. Like the example above, the image value must be stored in the app’s local directory.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn