What Is Isomorphic React
- Understanding Isomorphic React
- Setting Up Your Environment
- Creating a Simple Isomorphic React Application
- Benefits of Isomorphic React
- Conclusion
- FAQ
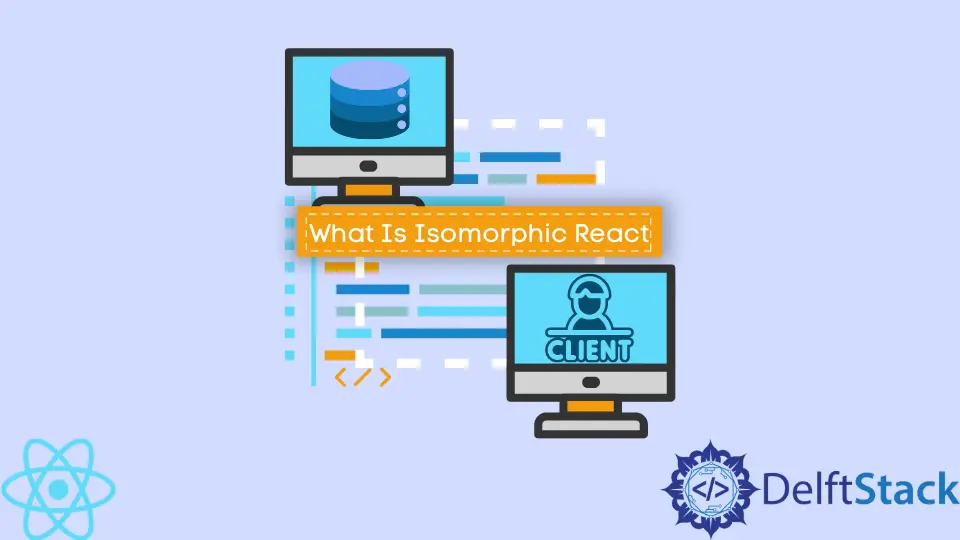
Isomorphic React, also known as Universal React, is an innovative approach that allows developers to run the same code on both the client and server sides. This means that the rendering of components can happen on the server, delivering an initial HTML response to the browser, while still allowing for dynamic interactions on the client side. This technique not only improves performance but also enhances SEO capabilities, making it a popular choice among web developers.
In this tutorial, we will dive deep into what Isomorphic React is, how it works, and why it is beneficial for modern web applications. Get ready to explore the intricacies of this powerful framework and learn how you can implement it in your projects.
Understanding Isomorphic React
Isomorphic React leverages the strengths of server-side rendering (SSR) and client-side rendering (CSR). By allowing JavaScript code to be executed on both the server and client, it provides a seamless user experience. When a user first accesses a webpage, the server sends a fully rendered HTML page. This means that the user can see content immediately without waiting for JavaScript to load and execute.
Once the page is loaded, React takes over, enabling dynamic interactions without requiring a full page refresh. This hybrid approach not only speeds up the initial load time but also improves SEO since search engines can crawl the fully rendered content.
To illustrate how Isomorphic React works, let’s look at a simple example where we create a basic React component that can be rendered on both the server and client.
Setting Up Your Environment
Before diving into the code, ensure you have Node.js and npm installed on your machine. These tools will help us manage dependencies and run our server. Once you have those set up, create a new directory for your project, navigate to it in your terminal, and run the following command to initialize a new Node.js project:
npm init -y
This will create a package.json
file where we can manage our dependencies. Next, install the required packages:
npm install express react react-dom
With these packages installed, we are ready to create our Isomorphic React application.
Creating a Simple Isomorphic React Application
Let’s create a simple React component and set up an Express server to render it. First, create a new file named server.js
. This file will contain our server-side code.
const express = require('express');
const React = require('react');
const ReactDOMServer = require('react-dom/server');
const app = express();
const App = () => (
<div>
<h1>Hello, Isomorphic React!</h1>
<p>This content is rendered on the server and sent to the client.</p>
</div>
);
app.get('/', (req, res) => {
const html = ReactDOMServer.renderToString(<App />);
res.send(`
<!DOCTYPE html>
<html>
<head>
<title>Isomorphic React Example</title>
</head>
<body>
<div id="app">${html}</div>
<script src="client.js"></script>
</body>
</html>
`);
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
In this code, we create an Express server that listens on port 3000. When a user accesses the root URL, the server uses ReactDOMServer.renderToString()
to render the App
component into a string of HTML. This HTML is then sent as a response to the client.
Output:
Server is running on http://localhost:3000
Next, let’s create the client.js
file to handle client-side rendering.
import React from 'react';
import { hydrate } from 'react-dom';
const App = () => (
<div>
<h1>Hello, Isomorphic React!</h1>
<p>This content is rendered on the server and sent to the client.</p>
</div>
);
hydrate(<App />, document.getElementById('app'));
In this client.js
file, we import the hydrate
method from react-dom
. This method takes the server-rendered HTML and attaches event handlers to it, allowing for a fully interactive experience on the client side.
Benefits of Isomorphic React
The advantages of using Isomorphic React are numerous. First and foremost, it significantly improves the performance of web applications. Since the server sends pre-rendered HTML to the client, users can see content almost instantly, enhancing user experience.
Additionally, Isomorphic React improves SEO. Search engines can easily crawl and index the fully rendered HTML, ensuring better visibility in search results. This is particularly beneficial for content-heavy websites or applications where search engine traffic is vital.
Moreover, code reusability is another key benefit. Developers can write components once and use them on both the client and server sides, leading to cleaner and more maintainable code. This not only speeds up development but also reduces the risk of bugs.
In summary, Isomorphic React offers improved performance, better SEO, and enhanced code reusability, making it an attractive choice for modern web development.
Conclusion
Isomorphic React represents a powerful approach to building web applications that deliver a seamless user experience while also optimizing performance and SEO. By allowing code to run on both the server and client, developers can create applications that are not only fast but also engaging. As web technologies continue to evolve, understanding and implementing Isomorphic React can provide a significant advantage in creating robust, scalable applications.
Whether you are building a small project or a large-scale application, considering Isomorphic React could be a game changer for your development process.
FAQ
-
what is isomorphic react?
Isomorphic React, or Universal React, allows the same code to run on both the server and client sides, enhancing performance and SEO. -
how does isomorphic react improve performance?
By rendering components on the server, Isomorphic React sends pre-rendered HTML to the client, enabling faster initial load times. -
can isomorphic react be used with other frameworks?
Yes, Isomorphic React can be integrated with frameworks like Next.js, which simplifies the process of server-side rendering. -
what are the challenges of using isomorphic react?
Challenges include increased complexity in code management and potential issues with client-side state synchronization. -
is isomorphic react suitable for all types of applications?
While it offers many benefits, Isomorphic React is particularly advantageous for content-heavy applications that require optimized SEO.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn