The hashRouter Component in React
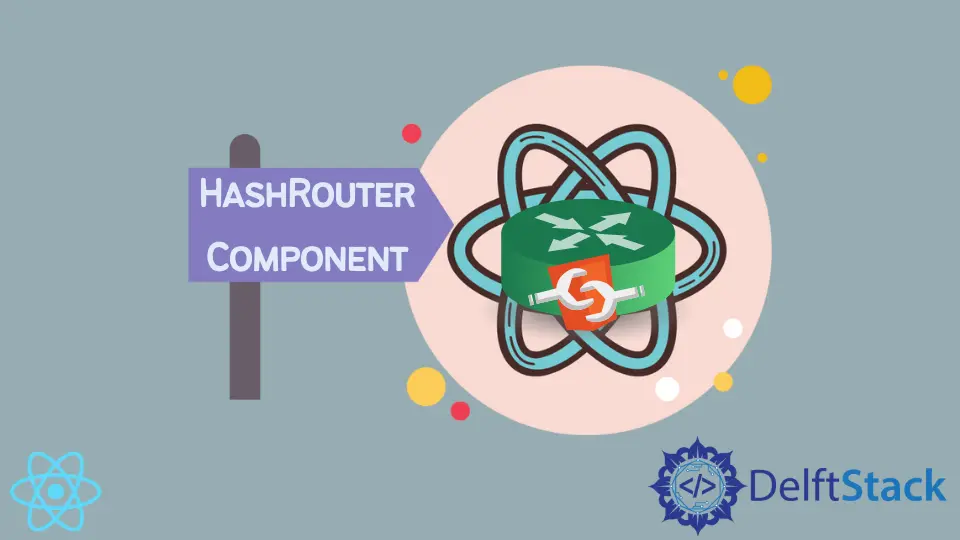
React is a library that lets you build modern, fast applications with stylish UI. Routing is one of the essential features of any user-facing application.
In React, we use a react-router
library to allow users to navigate our app by implementing the routing features.
the <HashRouter>
Component in React
<HashRouter>
is a sub-type of the <Router>
component that uses a hash value (available on window.location.hash
) to update the UI of the application based on changes to the URL. The component gets its name because it uses a hash symbol. Everything that follows this symbol will be ignored in the server request.
Because it uses a hash value to conditionally render components, <HashRouter>
supports old and new browsers. Client-side routing with <HashRouter>
is decoupled from server-side routing.
For example, if the server request is www.sample.com/#/category/home
, the final request received by the server will be www.sample.com
, and the server-side routing will render the page for www.sample.com
, not the entire URL, including the parts after the hash symbol.
Instead, you can use the <HashRouter>
component to change the UI based on the URL after the hash symbol. This is done on the client-side.
To learn more about when to use the <HashRouter>
vs. <BrowserRouter>
, read this article.
To better understand how to use the <HashRouter>
component in practice, let’s look at this basic example:
import "./styles.css";
import { HashRouter, Link } from "react-router-dom";
export default function App() {
return (
<HashRouter basename="/">
<div className="App">
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
<Link to="/category1">hello</Link>
</div>
</HashRouter>
);
}
We imported two custom components from the react-router-dom
library: <HashRouter>
and <Link>
. We wrapped the entire application with the <HashRouter>
custom component.
We added a <Link>
component in the container that takes the user to the "/category1"
path from the current URL.
Check out this live demo to see how it works in practice.
As you can see, the <HashRouter>
component has a basename
attribute set equal to simply "/"
. For this reason, the homepage of this app is https://bweuok.csb.app/#/
.
Clicking the link will take you to the https://bweuok.csb.app/#/category1
path.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn