Difference Between Route Exact Path and Route Path
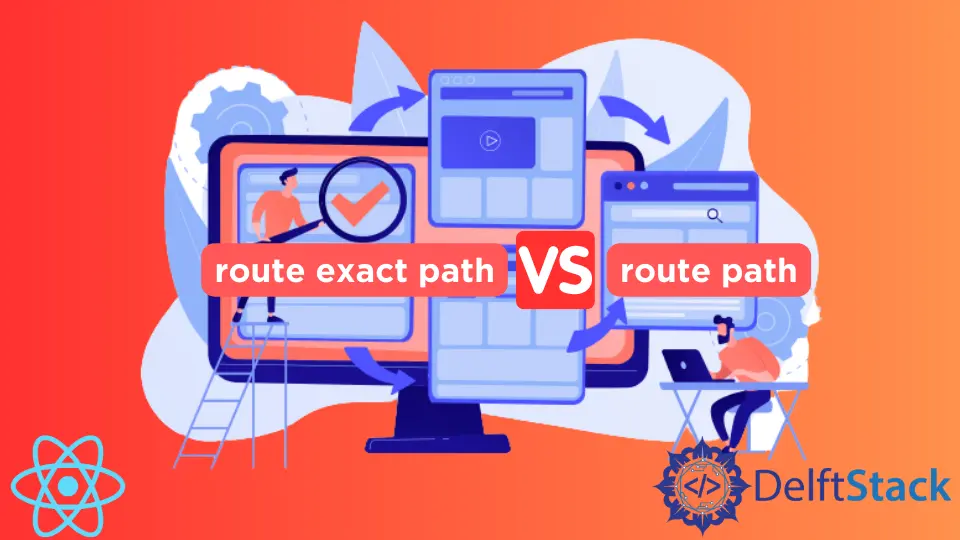
The react router
is a way to create multi-page applications in React; when applied, it creates the functionality that allows users to click on a link that takes them to a new page.
When we create two or more links within a component, things become tricky, and we need to improvise.
Difference Between Route Exact Path and Route Path
When we have two or more links within a component and use route path
, the page will render the items on both links if we only want to render the second link. But when we use the route exact path
, the page only renders the details in the second link.
Let us look at the example below.
First, we have created a new React project; next, we will navigate into the project folder in our terminal and install react router
; we will use npm install react-router-dom@5.2.0
.
Then we will put some codes inside our App.js
file like below.
Code Snippet - App.js
:
import './App.css';
import React, {Component} from 'react';
import {BrowserRouter as Router} from 'react-router-dom';
import Route from 'react-router-dom/Route';
class App extends Component {
render() {
return (
<Router>
<div className='App'>
<Route path='/' render={
() => {
return (<h1>Welcome Home</h1>);
}
} />
<Route path='/about' render={
() => {
return (<h1>About</h1>);
}
} />
</div>
</Router>
);
}
}
export default App;
Output:
When we run the app, we see "Welcome Home"
on the page, but when we try to navigate to the "about"
page with this address, "localhost:3000/about"
, we see that the page loads both routes and we see both "Welcome Home"
and "About"
.
This is because React reads the URL from the "/"
since we did not specify otherwise in our code.
But with the help of the exact path
, we can specify what we want React to read, so we do this in our code.
Code Snippet - App.js
:
import './App.css';
import React, {Component} from 'react';
import {BrowserRouter as Router} from 'react-router-dom';
import Route from 'react-router-dom/Route';
class App extends Component {
render() {
return (
<Router>
<div className='App'>
<Route exact path='/' render={
() => {
return (<h1>Welcome Home</h1>);
}
} />
<Route exact path='/about' render={
() => {
return (<h1>About</h1>);
}
} />
</div>
</Router>
);
}
}
export default App;
Output:
We add the exact path
to both components and see that when we go to the "About"
, it renders only the "about"
page.
Conclusion
Without the exact path
function, React developers will constantly need to create separate components for each link; this will lead to codes getting cluttered, website rendering will be slower, not to mention it will be a rigorous and repetitive exercise.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn