How to Disable Buttons in React
- Disable Button in React
- Disable Button After Button Click in React
- Disable Button When Input Field Is Empty and Enable When User Type in Input Field
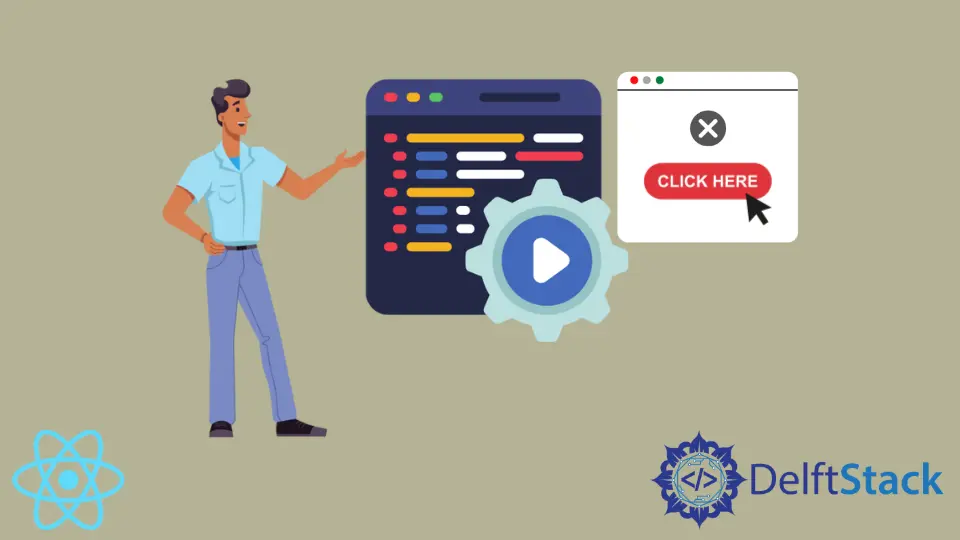
We will introduce how to disable the button in react.js using a disabled prop to our button
element.
We will also introduce the method to disable the button after we have clicked on it.
We will also introduce the method to use disable the button
in forms to stop users from clicking on buttons before entering data in input fields.
Disable Button in React
To simply disable the button, we can use the disabled prop
in our button element and set its value to true
. It is the simplest way to disable any button in react.
Example Code:
# react.js
import React from "react";
function Disable(){
return <button disabled={true}>I am Disabled!</button>
}
export default Disable
Output:
You can see in the above output preview that our button is disabled.
Disable Button After Button Click in React
If we want to disable our button after clicking on it, We can disable it by using react’s state. We will set the button’s disable
state to false
on load and add the onClick
function in our button element, which will set the button’s disable
state to true
. So, When a user clicks on the button, it will change its disable
state to true
.
Example Code:
# react.js
import React from "react";
function DisableAfterClick() {
const [disable, setDisable] = React.useState(false);
return (
<button disabled={disable} onClick={() => setDisable(true)}>
Click to Disable!
</button>
);
}
export default DisableAfterClick
Output:
You can see in the above output preview that our button is disabled. But there is no way to enable the button after it is disabled.
Disable Button When Input Field Is Empty and Enable When User Type in Input Field
If we want to enable a disabled button when a user enters text in the input field and disable it when the user clears the input field, we can use a disabled
function. So, When the user types something in the input field, the disabled
function will get a false
value from !text
, which will change the state
of a button from disabled
to enabled
. And when the user clears the input field, !text
will return true
and change the state
of the button to disabled
.
Example Code:
import React, { useState } from "react";
function EnableOnInputChange() {
const [text, enableButton] = useState("");
const handleTextChange = (event) => {
enableButton(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
alert(`Your state values: \n
text: ${text} \n
You can replace this alert with your process`);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Enter Text</label>
<input
type="text"
name="text"
placeholder="Enter Text"
onChange={handleTextChange}
value={text}
/>
</div>
<button type="submit" disabled={!text}>
Enable
</button>
</form>
);
}
export default EnableOnInputChange
Output:
You can see in the above output, When we enter in Input field button is enabled, and when we clear, the input field button is disabled again.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn