How to Create Multiple Page App Using React
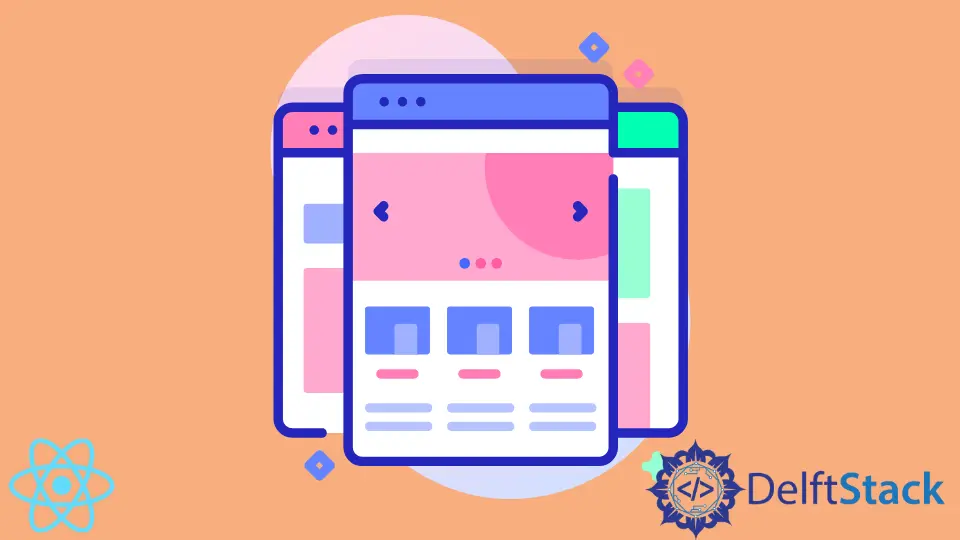
This article will demonstrate creating a multiple-page web application using React, a JavaScript library for building US (user-interfaces).
Create Multiple Page Application in React
First, we create a new project in the terminal by typing npx create-react-app new-project
, then we navigate to the app folder and start the app using npm start
.
In the src
folder of the project, we create the following files:
Home.js
About.js
Projects.js
Contact.js
Then we open the App.js
file in the text editor and paste this code below.
Code Snippet - App.js
:
import React from "react";
import logo from "./logo.svg";
import "./App.css";
import Home from './Home';
import Projects from './Projects';
import Contact from './Contact';
import About from './About';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import { Link } from "react-router-dom";
import NavBar from './NavBar';
function App() {
return (
<div className="App">
<NavBar />
<Routes>
<Route exact path="/" element={<Home />} />
<Route exact path="/about" element={<About />} />
<Route exact path="/projects" element={<Projects />} />
<Route exact path="/contact" element={<Contact />} />
</Routes>
</div>
);
}
export default App;
In the App.js
, we will import Route
and Link
from the react-router-dom
and create a navbar.js
to enable us to navigate through each web page. The following are the snippets of the created files in the project mentioned above.
Code Snippet - navbar.js
:
import React from 'react'
import {Link} from 'react-router-dom'
function NavBar(){
return(
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/projects">Projects</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
);
}
export default NavBar;
Code Snippet - Home.js
:
import React from 'react'
function Home(){
return(
<div>
<h1>Welcome to my website!</h1>
</div>
)
}
export default Home;
Code Snippet - About.js
:
import React from 'react'
function About(){
return(
<div>
<h1>About Me!</h1>
</div>
)
}
export default About;
Code Snippet - Rpojects.js
:
import React from 'react'
function Projects(){
return(
<div>
<h1>My Projects</h1>
</div>
)
}
export default Projects;
Code Snippet - Contact.js
:
import React from 'react'
function Contact(){
return(
<div>
<h1>Contact Me!</h1>
</div>
)
}
export default Contact;
Installing and Deploying the React Router
After we do everything above, we will install the react-router -dom
. Open the terminal in the project folder, then we type in, npm install --save react-router-dom
.
After installation, we head over to the index.js
file, import the BrowserRouter
from react-router-dom
and wrap the BrowserRouter
in the ReactDOM.render
.
Code Snippet - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { BrowserRouter } from "react-router-dom";
import { Route, Routes } from "react-router-dom";
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
When all is done, we should be able to click on each link and switch pages instantly, like the output below.
Output:
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn