Axios GET Header in React Native
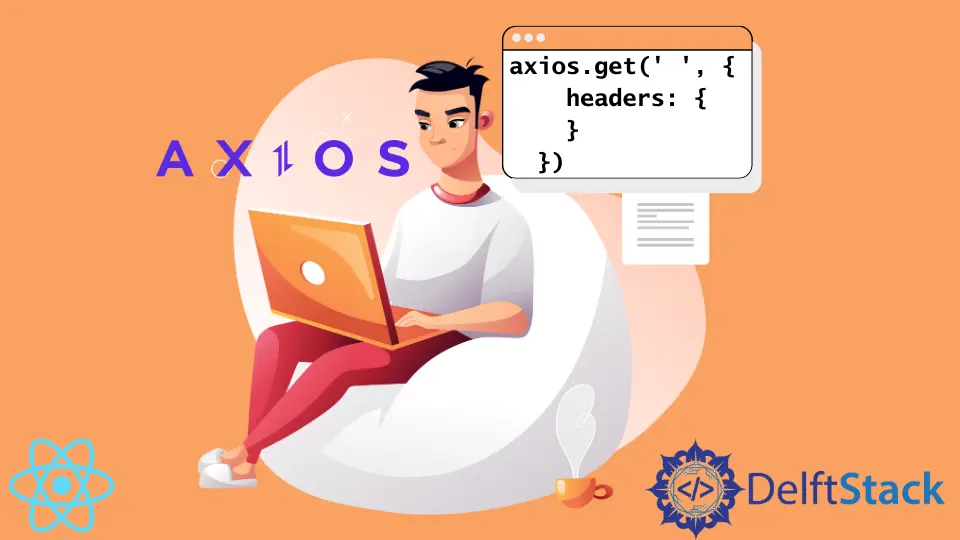
Axios
is mainly used for making HTTP requests. The most exciting thing about this is that it can make both XMLHttpRequest
and HTTP requests.
There are two mostly used HTTP requests available. These are the GET
and POST
.
Some other request methods are available, like PATCH
, DELETE
, etc. But in this article, we will only discuss the GET
method.
The method axios.get()
is mainly used for performing GET
requests from a React-Native app. It takes a URL as a parameter.
When you successfully make a GET
request, you will get a response. You can use an Authorization
header when making the request.
In this article, we will see how we can use the axios.get()
method with the Authorization
header in a React-Native app. Also, we will see an example and explanation to make the topic easier.
Use Axios GET
Method With Authorization
Header in React-Native
In our example below, we will demonstrate how we use the axios.get()
method in our React-Native app. The code for our example will be as follows:
// Import necessary packages
import axios from 'axios';
export default function App() {
// Creating an axios GET request
axios.get('https://example.com', {headers: {'Authorization': 'My token'}})
.then((response) => {
console.log(response.data); // Processing the response text
})
}
The purpose of each block of codes regarding the steps shared above in the example is left as comments.
In the example above, we are getting the data of example.com
by using axios.get('https://example.com')
, and after that, we receive the data stored as response
.
Lastly, we print the received data in the console by console.log(response.data)
. We also applied an Authorization
header like the one below.
headers: {'Authorization': 'My token'}
You will get the below output on your terminal console when you run the app.
Please note that the code shared above is created in React-Native
, and we used the Expo-CLI
to run the app. Also, you need the latest version of Node.js
.
Install it if you don’t have Expo-CLI
in your environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn