How to Add Favicon to React Applications
- Understanding the Importance of a Favicon
- Setting Up Your Favicon
- Method 1: Adding Favicon Using Webpack
- Method 2: Using React Helmet for Dynamic Favicon
- Conclusion
- FAQ
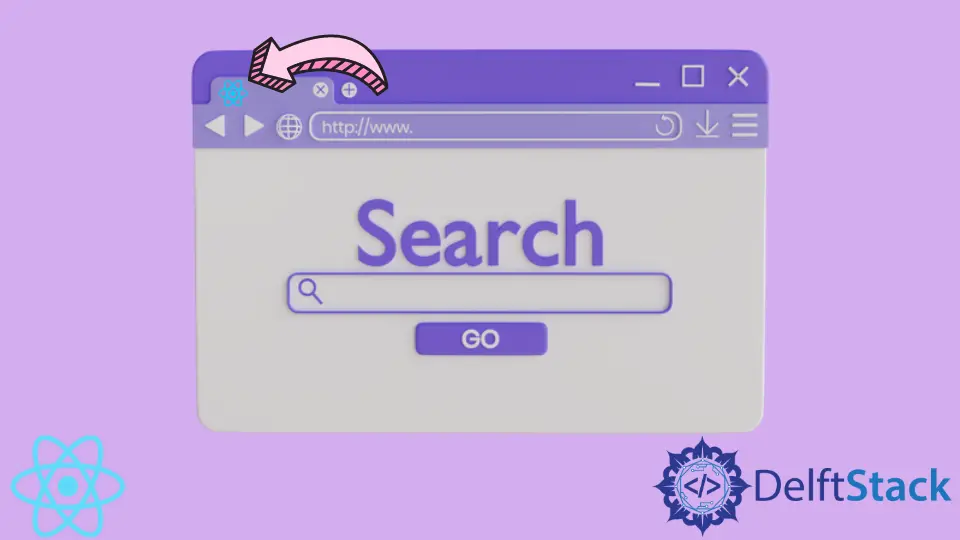
Favicon is an important but often overlooked feature in web applications. A favicon, the small icon that appears in the browser tab next to your website title, plays a crucial role in branding and user experience. If you’ve built a React application and want to enhance its professional appeal, adding a favicon is a simple yet effective step. In this guide, we will explore how to set up a favicon in React applications using Webpack. By following these steps, you will not only improve the visual identity of your app but also ensure it stands out in users’ browser tabs. Let’s dive into the process of adding a favicon seamlessly.
Understanding the Importance of a Favicon
Before we jump into the technical steps, it’s essential to understand why a favicon matters. A well-designed favicon helps users recognize your site more easily, especially when they have multiple tabs open. It adds a layer of professionalism and can even improve click-through rates from bookmarks or search results. In short, a favicon is a small detail that can make a significant impact on the overall user experience.
Setting Up Your Favicon
To add a favicon to your React application, you’ll need to follow a few straightforward steps. First, you need to create a favicon file. This is typically a small square image, usually 16x16 pixels or 32x32 pixels, saved in .ico format. You can use various online tools to convert images into the appropriate favicon format. Once you have your favicon ready, you can proceed with the following methods to integrate it into your React app.
Method 1: Adding Favicon Using Webpack
If you’re using Webpack to bundle your React application, you can easily add a favicon by following these steps:
- Place your favicon.ico file in the
public
directory of your React project. - Modify the
index.html
file located in thepublic
directory to include a link to the favicon.
Here’s how your index.html
should look:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<title>Your React App</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
In this snippet, the <link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
line is crucial as it tells the browser where to find your favicon. %PUBLIC_URL%
is a placeholder that Webpack replaces with the correct path during the build process.
Output:
Favicon successfully linked to your React application.
By following these steps, your favicon will appear in the browser tab when users visit your application. This method is straightforward and integrates well with the React ecosystem, especially if you’re already using Webpack for your project.
Method 2: Using React Helmet for Dynamic Favicon
If you’re looking for a more dynamic approach, especially if your application has different pages or themes, using React Helmet can be a great option. React Helmet allows you to manage your document head, including the favicon, dynamically.
First, you’ll need to install React Helmet. You can do this by running:
npm install react-helmet
Once installed, you can use it in your components like this:
import React from 'react';
import { Helmet } from 'react-helmet';
const MyComponent = () => {
return (
<div>
<Helmet>
<link rel="icon" href="/favicon.ico" />
<title>Your Dynamic Title</title>
</Helmet>
<h1>Welcome to My React App</h1>
</div>
);
};
export default MyComponent;
In this example, the Helmet
component is used to set the favicon dynamically. You can change the favicon based on the component or page being viewed, enhancing the user experience.
Output:
Dynamic favicon set for the component.
Using React Helmet is particularly beneficial for single-page applications (SPAs) where the content changes frequently. It provides flexibility and control over how your application is presented in the browser.
Conclusion
Adding a favicon to your React application is a simple yet impactful way to enhance your web presence. With methods like integrating it directly through Webpack or utilizing React Helmet for dynamic changes, you can easily implement this feature. A well-designed favicon not only improves user experience but also contributes to your brand identity. So, take a moment to create a favicon for your React app and make that first impression count!
FAQ
-
How do I create a favicon?
You can create a favicon using online tools that convert images to .ico format or design one using graphic design software. -
Can I use different favicons for different pages?
Yes, by using React Helmet, you can set different favicons for different components or pages in your React application. -
What size should my favicon be?
The standard size for a favicon is 16x16 pixels or 32x32 pixels, but you can create larger sizes for better resolution on high-DPI displays. -
Do I need to clear my browser cache to see the favicon?
Sometimes, yes. Browsers cache favicons, so you may need to clear your cache or do a hard refresh to see changes. -
Is it necessary to have a favicon for my website?
While not mandatory, having a favicon enhances your website’s professionalism and improves user experience.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn